Trees in data structures are a type of non-linear data structure that illustrates a hierarchical relationship between objects. It’s composed of nodes, with distinctive root and leaf nodes, interconnected via edges. Different forms of trees include binary and AVL trees. They enable efficient data sorting, retrieval, and facilitate operations like searching due to their branch-like structure.
Main Points
- Trees in data structures are hierarchical systems with a single root node and multiple child nodes, forming a non-linear, branching structure.
- Key terminologies in trees include nodes, leaf nodes, root nodes, edges, and parent-child relationships.
- Varieties of trees include binary trees, AVL trees, and B-Trees, each with unique characteristics and applications.
- Trees in data structures operation involve root node representation, subtrees, tree traversal, and depth-first search, benefiting from their non-linear structure.
- Practical applications of tree structures include file systems, sorting and searching operations, priority queues, database indexing, and compiler design.
Understanding Trees in Data Structures
In order to fully grasp the concept of trees in data structures, one must first recognize its hierarchical nature, with a single root node from which multiple child nodes branch out, forming a non-linear system that efficiently represents relationships and hierarchies. This branching structure is a distinctive feature of tree data structures and is pivotal in creating a hierarchical structure of data. Each node in a tree can have zero or more child nodes, allowing for complex and varied organization of data.
The depth of a node is another key aspect of tree data structures. The depth signifies the length of the path from the root to a particular node, providing insight into the node’s position in the hierarchical structure. This attribute is critical in several algorithmic computations and operations performed on trees.
Trees, as non-linear data structures, are paramount in implementing algorithms like binary search, and in effectively modeling hierarchical relationships. The hierarchical structure and depth attributes make trees an excellent choice for organizing data in a manner that facilitates efficient search, insertion, and deletion operations. Hence, understanding tree data structures is fundamental to mastering data organization and manipulation.
Key Terminologies for Trees in Data Structures
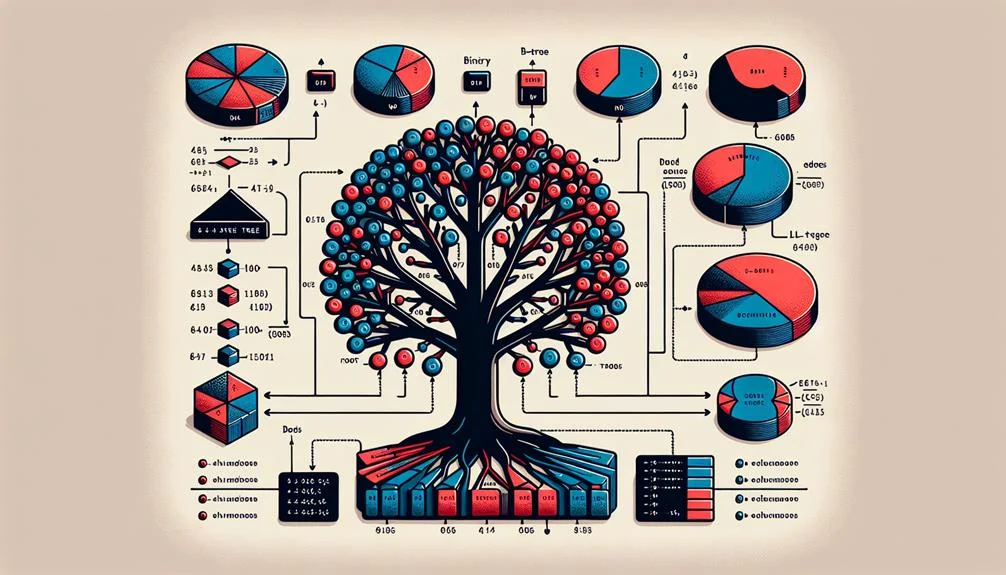
To explore further into the complexities of trees in data structures, it’s crucial to acquaint ourselves with the essential terminologies, such as nodes, leaf nodes, root nodes, and edges.
Nodes are fundamental units of a tree data structure, containing data and providing connections to other nodes. They are classified into leaf nodes, internal nodes, and a root node. Leaf nodes, or ‘leaves‘, are the nodes at the endpoints of the tree. These nodes have no child nodes attached to them.
Internal nodes are those with at least one child node, acting as a bridge in the hierarchical relationship of the tree. The root node is the topmost node in the tree structure, the origin from which all other nodes emanate. It is the only node that doesn’t have a parent node, thereby making it unique.
Edges are the lines that connect nodes in the tree, forming parent-child relationships. These relationships are the essence of the tree structure, allowing for the organization and traversal of data in a hierarchical manner. Understanding these key terminologies provides a firm foundation for comprehending the intricacies of tree data structures.
Various Types of Trees in Data Structures?
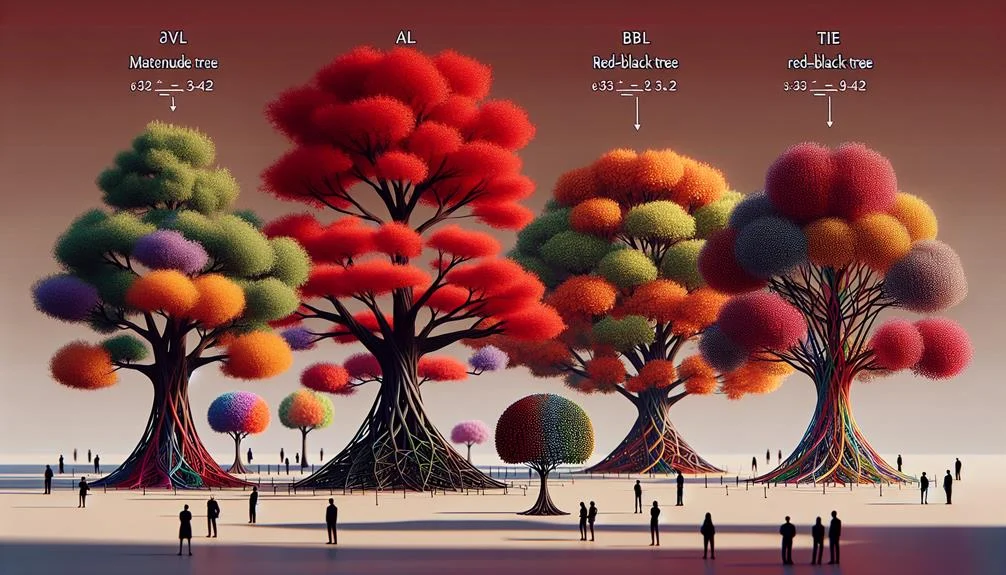
In our ongoing exploration of trees in data structures, we now turn our attention to the various types of trees, commencing with Binary Trees. These trees, characterized by a node structure allowing at most two children, provide a foundation for efficient search and traversal operations.
We will then progress to a thorough analysis of AVL Trees, before moving onto the intricate multiway structure of B-Trees.
Binary Trees Basics
Investigating binary trees, a fundamental type of tree data structure, we come across nodes that have at most two children, leading to several unique forms such as full binary trees, complete binary trees, binary search trees, and balanced binary search trees.
- Full binary trees contain nodes with either zero or two children. This structure eliminates the instance of a node having only one child.
- Complete binary trees are binary trees filled from left to right, ensuring efficient use of space.
- Binary search trees (BST) provide effective search capabilities in O(log n) time, leveraging the property of nodes’ left child being less than the parent node and the right child being greater.
- Balanced binary search trees maintain equilibrium in the tree structure, thereby enhancing search efficiency, especially during insertions and deletions. These trees are essential for implementing efficient traversal algorithms in data structures.
Understanding AVL Trees
Exploring the domain of self-balancing binary search trees, we encounter AVL trees, a distinct and effective type of height-balanced binary search tree named after its creators, Adelson-Velsky and Landis.
AVL trees’ unique characteristic lies in their balance factor, which guarantees that the height difference between the left and right subtrees of any node is at most 1. This balance factor is maintained through the use of rotations triggered by insertions and deletions.
Consequently, AVL trees offer efficient search operations with a time complexity of O(log n), owing to their self-balancing nature. Hence, AVL trees provide a robust and efficient solution in applications requiring optimally performant search algorithms.
Exploring B-Trees in Data Structures?
As we look into self-balancing tree data structures, we discover B-Trees, a highly efficient system primarily employed in databases and file systems to maintain sorted data with utmost proficiency.
- B-Trees in Data Structures are self-balancing and disk-based data structures, specifically designed to minimize disk I/O operations for large-scale storage systems.
- These trees have a high fan-out factor, accommodating a variable number of child nodes per node, which optimizes search operations.
- Their structure is ideal for efficient storage and data retrieval, ensuring quick access times in scenarios where data is frequently accessed.
- B-Trees in Data Structures are primed for maintaining sorted data, making them an essential component in the domain of databases and file systems.
Representation and Operations of Trees in Data Structures
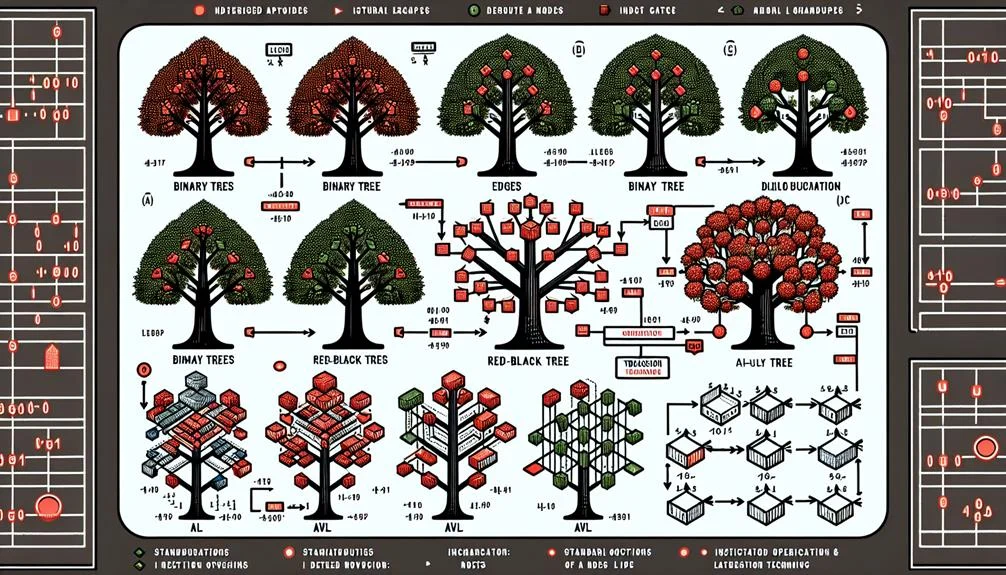
In data structures, tree representation and operations, characterized by a root node and potentially numerous subtrees, play a pivotal role in data organization and manipulation. Unlike linear data structures such as a linked list, trees allow for the dynamic handling of hierarchical data, providing an efficient approach to searching and sorting data.
The tree structure can be visualized as an adjacency list, with the root node at the top and the leaf nodes, or the end nodes of each subtree, at the bottom. The subtrees are defined by their parent-child relationships, with each child node potentially branching into more subtrees.
The process of tree traversal involves visiting each node in a specific order: Preorder, Inorder, Postorder, Level order, or Depth First Search. This operation is essential for efficient searching within the tree.
Trees can be classified into different types based on their structure and properties. Two common types are complete binary trees, where each node has zero, one, or two children, and balanced binary trees optimized for binary search operations. Understanding these operations and representations is foundational for effective data manipulation.
Non-Linearity of Trees in Data Structures
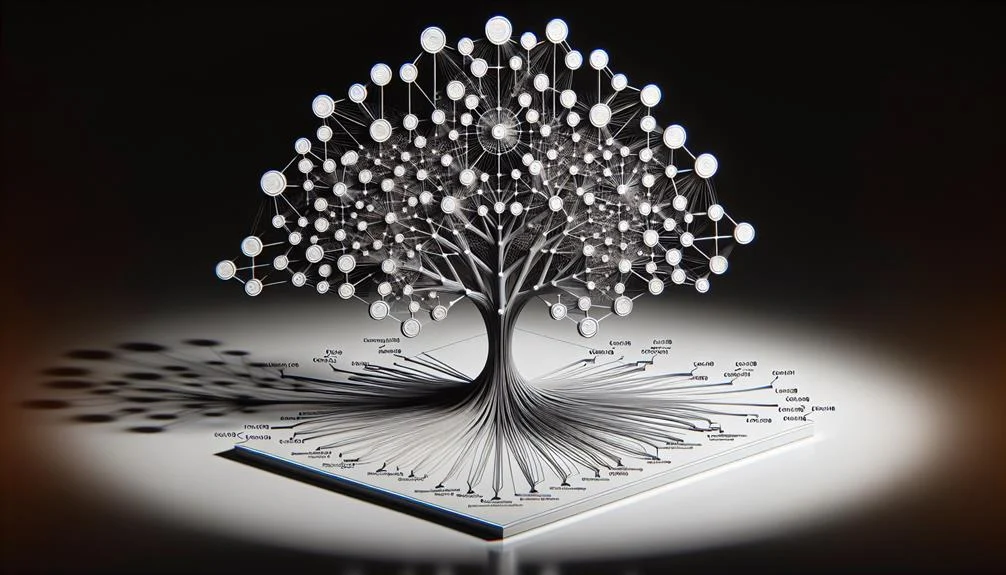
The non-linearity of tree structures in data operations sets them apart from their linear counterparts, such as arrays and linked lists. This characteristic, manifesting in a hierarchical relationship among nodes, results in a versatile data structure capable of organizing data in an efficient, structured manner.
The branching nature of tree structures makes them a powerful tool for modelling complex relationships and data sets.
Understanding Tree Non-Linearity
While linear data structures such as arrays or linked lists organize data in sequential order, trees, on the other hand, are renowned for their non-linearity, where nodes are interconnected in a hierarchical manner rather than a linear progression. This non-linearity facilitates branching and multiple paths, enabling complex relationships amongst the nodes.
- Non-linearity: Trees, unlike linear data structures, do not follow a sequential order, allowing for various paths and branching.
- Hierarchical Interconnections: The nodes in tree data structures are connected in a hierarchical fashion, promoting efficient representation of data.
- Complex Relationships: The non-linear structure allows for intricate relationships between nodes.
- Advantageous Characteristics: Understanding the non-linearity in tree structures reveals their unique characteristics and advantages, including efficient representation and organizational flexibility.
Branching in Tree Structures
Building upon our understanding of non-linearity in tree structures, we now turn our focus to the concept of branching. This key feature contributes to these data structures’ hierarchical and non-linear nature. Branching allows each node to have multiple children nodes, forming a binary search structure capable of efficiently organizing data and representing complex relationships.
This ability to branch out and form different levels of hierarchy, is inherent in tree structures and essential in modeling diverse subtree configurations and parent-child connections.
Structure Element | Role in Branching |
---|---|
Nodes | Serve as branching points |
Children | Result of branching from parent nodes |
Hierarchical Organization | Facilitated by branching |
Representation | Enhanced through branching |
Binary Search | Enabled by branching |
This provides a detailed, technical, and analytical understanding of the role of branching in tree structures.
Implications of Tree Non-Linearity
Examining the implications of tree non-linearity in data structures, we find itessential in establishing hierarchical relationships between nodes, effectively representing parent-child connections, and facilitating efficient search and traversal operations. Non-linear tree structures provide a dynamic way to organize diverse data, which is crucial in many computational scenarios.
- Hierarchical relationships: Tree structures inherently define a hierarchy, allowing for complex parent-child relationships and multiple children nodes.
- Branching structures: Branching, a consequence of non-linearity, leads to diverse and dynamic data organization.
- Efficient searching: The non-linear structure of trees enables efficient searching, crucial in database operations.
- Traversal operations: Non-linearity permits various traversal operations, fundamental for algorithms that require exploration of data elements.
Properties and Characteristics of Trees in Data Structures?
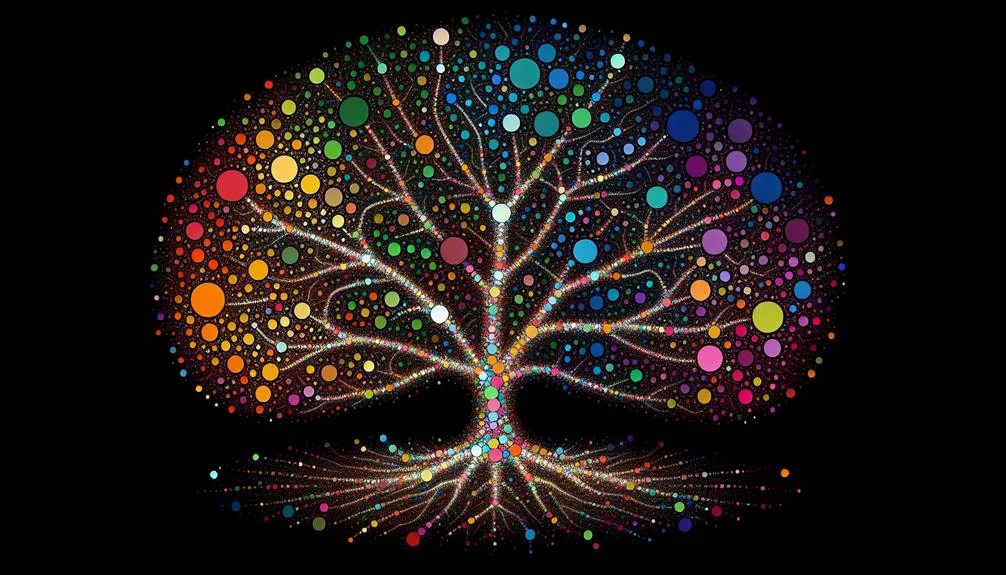
What are the inherent properties and distinguishing characteristics of trees in data structures? As non-linear structures, trees are anchored by a root node, branched into internal nodes, and terminated by leaf nodes. This hierarchical structure is fundamental for data organization, facilitating efficient searching and sorting operations.
A tree’s root node is its topmost point, with no parent but potentially multiple children. Internal nodes, distinguished by having at least one child, form complex relationships representing data. Leaf nodes, the endpoints, have no children.
The non-linearity of trees provides a significant advantage. It allows data to branch out, facilitating quicker access during searching or sorting. This contrasts linear structures where data elements have a sequential relationship.
Incorporating these properties into a table:
Properties of Trees | Characteristics |
---|---|
Root Node | Topmost point with potentially multiple children |
Internal Nodes | Have at least one child, enabling complex data relationships |
Leaf Nodes | Endpoints with no children |
Practical Applications of Trees in Data Structures
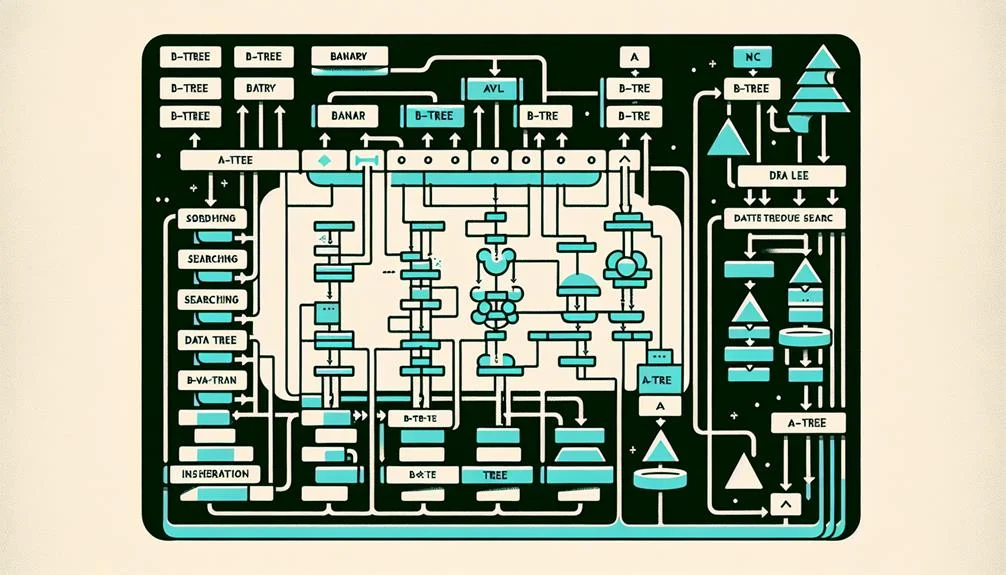
Numerous practical applications have been devised to harness the inherent hierarchy and non-linearity of tree structures, ranging from file system organization to compiler design. These structures offer a systematic method for managing data, enabling efficient operations in various domains.
- File Systems: In file systems, tree structures are used to organize directories and files hierarchically. This enables efficient file searches and management, enhancing system performance.
- Data Sorting and Searching: Binary Search Trees (BSTs) are commonly employed for sorting and searching operations. By maintaining a specific order, BSTs guarantee peak performance in data retrieval tasks.
- Priority Queues: Heaps, a type of tree structure, are utilized for implementing priority queues, ordering elements based on their priority levels for efficient execution.
- Database Indexing: B-Trees and B+ Trees, known for their balanced nature, are extensively used for indexing in databases. By splitting data into smaller, manageable sub-trees, they ensure quicker access to required data.
- Compiler Design: Syntax Trees play a pivotal role in compiler design, parsing, and evaluating programming language syntax. By representing grammatical constructs of a language, they aid in the correct interpretation of code.
Thus, tree structures, with their hierarchical and non-linear nature, find extensive applications in contemporary data management and processing tasks.
Pros and Cons of Trees in Data Structures
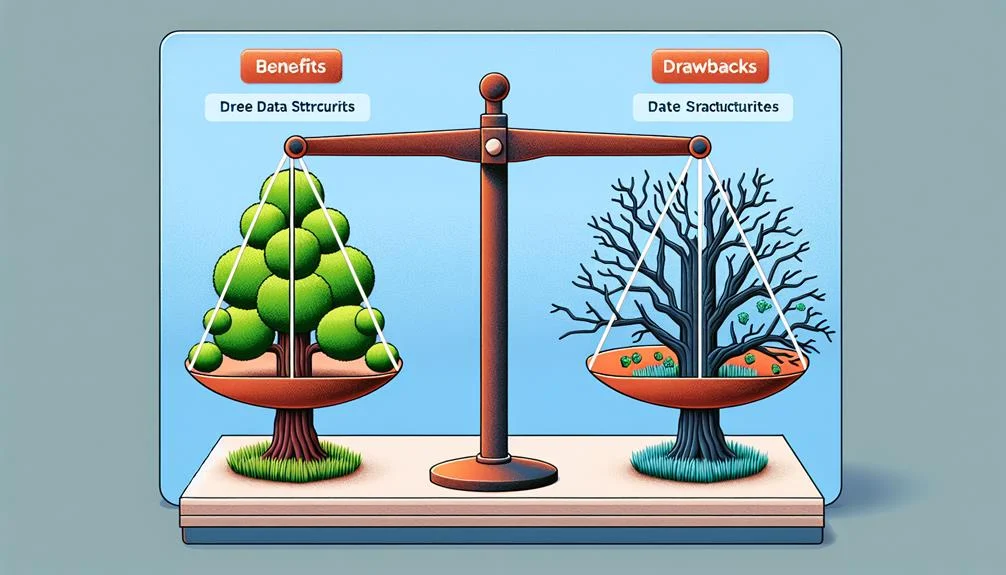
While the applications above showcase the vast utility of tree structures, it is equally important to critically assess the pros and cons of these data structures to comprehend their functionality and potential limitations fully.
One of the critical advantages of trees in data structures lies in their hierarchical structure. This structure allows for efficient searching and sorting capabilities. For example, binary search trees enable data to be accessed, searched, inserted, and deleted in logarithmic time, providing a significant speed advantage over linear data structures like arrays and linked lists.
Tree data structures facilitate dynamic insertion and deletion of elements, making them adaptable to changing data sets. This adaptability is pivotal in applications dealing with growing or shrinking data sets, where the ability to reorganize data efficiently is essential.
Tree structures are not without their disadvantages. A significant drawback is the potential for unbalanced structures. This imbalance can lead to suboptimal performance, as operations that should be relatively fast become slower. For instance, in severely unbalanced trees, operations can degrade to linear time complexity, negating trees’ efficiency benefits.
Conclusion
Trees in data structures serve as a potent tool in computer science, providing efficient solutions to intricate problems. Like a well-organized library, they store and retrieve information in a structured, hierarchical manner, ensuring peak performance.
However, their non-linear nature and complexity in implementation pose notable challenges. Yet, their diverse applications, ranging from database management to AI, underscore their integral role in advancing the dynamic field of data management and computation.