Data structures are essential frameworks in computer science used for efficient data organization, storage, and management. Core types include arrays, linked lists, stacks, queues, trees, and graphs, each having unique functions and applications. Arrays facilitate quick access with their contiguous memory structure, whereas linked lists operate via pointers, allowing flexible data arrangement. Stacks follow the Last In, First Out principle, contrary to queues, which use First In, First Out. Trees and graphs offer advanced data manipulation capabilities. Profound comprehension of these structures, their intricacies, and utilizations helps optimize memory use and enhance operational efficiency.
Main Points
- Data structures are fundamental ways of storing and organizing data in a computer for efficient use.
- Basic types of data structures include arrays, linked lists, stacks, queues, trees, and graphs.
- The choice of data structure depends on the data handling needs, such as quick access, flexible organization, or memory optimization.
- Some data structures like arrays offer quick retrieval and efficient storage, while others like linked lists allow dynamic memory allocation.
- Specialized data structures like heaps and hashing implement advanced techniques for data manipulation and quick access.
Understanding Basic Data Structures
To understand basic data structures, it is vital to grasp the fundamental concepts of arrays, linked lists, stacks, queues, trees, and graphs. Each offers unique advantages for data storage and manipulation, thereby playing an indispensable role in efficient data organization and processing.
Linked lists provide flexible data organization by connecting elements through pointers. This structure allows for dynamic memory allocation – a pivotal feature when the volume of data is unpredictable. Stacks, another data structure, operate on the Last In, First Out (LIFO) principle. This feature allows for efficient data manipulation, particularly in scenarios where the most recently added data is of utmost significance.
Queues adhere to a First In, First Out (FIFO) order. This characteristic guarantees orderly data processing and is particularly beneficial in situations where the sequence of data matters. Although arrays will be discussed in detail later, it is worth mentioning that they store elements in contiguous memory locations, facilitating quick data access.
Detailed Overview of Arrays
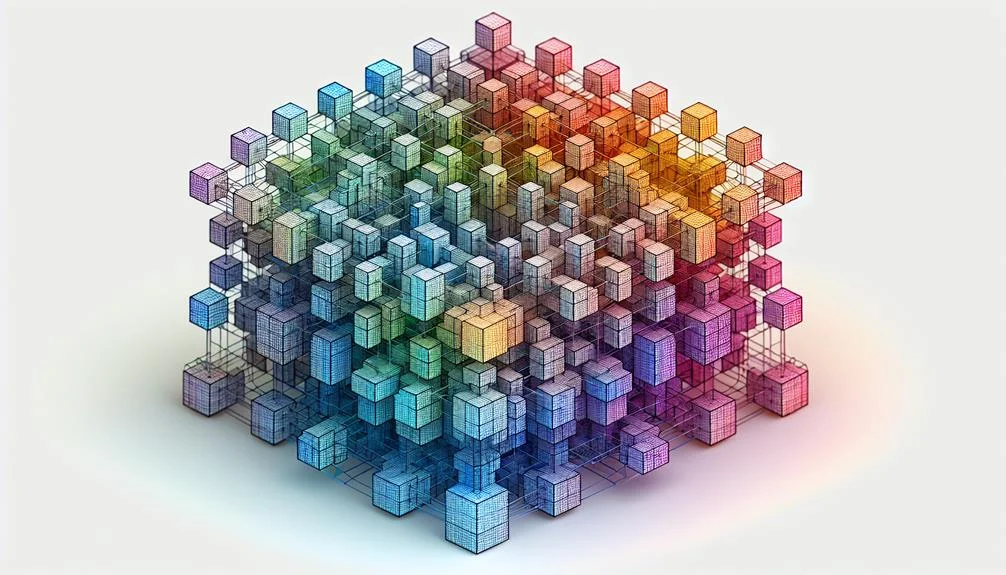
In data structures, arrays emerge as a fundamental tool characterized by their ability to store elements of the same data type in contiguous memory locations, thereby offering direct, efficient access to elements through index positions.
Arrays exhibit several key features that contribute to their effectiveness:
- Fixed Size: Arrays are defined with a fixed size during initialization, which cannot be altered later. This characteristic is advantageous when the number of elements is known in advance.
- Efficient Retrieval: Arrays offer efficient data retrieval due to their structure. Each element in an array can be accessed directly via its index, resulting in a constant time complexity of O(1) for both read and write operations.
- Versatile Data Storage: Arrays can be one-dimensional or multi-dimensional, providing adaptable and versatile data storage solutions. Multi-dimensional arrays further enhance the ability to represent complex data structures.
- Contiguous Memory: The elements of an array are stored in contiguous memory locations, facilitating faster access and efficient usage of memory.
Exploring Linked Lists
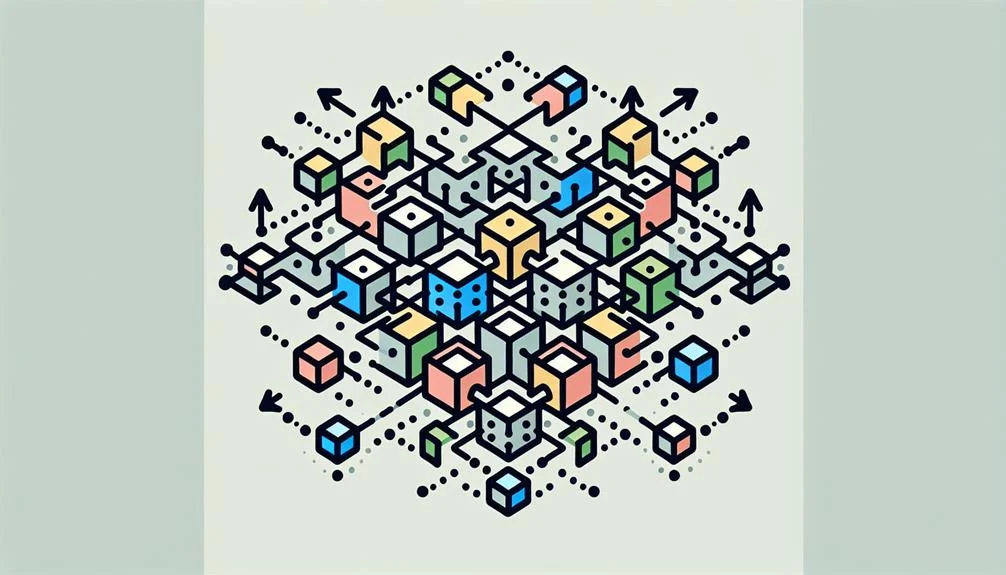
At the core of understanding linked lists is grasping the concept of nodes, the building blocks of this structure, each containing data and a reference to the next node sequentially.
This structure offers significant advantages, particularly with regards to dynamic memory allocation and efficient manipulation of data, making it a common choice in applications that require frequent data adjustments.
Understanding Linked Lists Basics
To comprehend the foundational concepts of linked lists – a quintessential linear data structure, it is essential to understand that they comprise nodes interconnected through pointers, each carrying data and a reference to the subsequent node in the sequence.
- Linked lists are dynamic, therefore the size can be adjusted during run-time, unlike arrays.
- They can be singly linked, where each node points only to the next node, or doubly linked, with each node pointing to both the next and the previous nodes, enhancing backward traversal.
- Efficient operations such as insertions and deletions merely require reconfiguring the pointers, sidestepping the need to shift elements as in arrays.
- Traversal through a linked list commences from the head node, ending at the tail node, following the pointers.
Utilizing Nodes in Lists
Exploring the intricacies of linked lists, it becomes evident that the efficient utilization of nodes in these lists is pivotal, providing dynamic memory allocation, non-contiguous data storage, and forming the backbone for more advanced data structures.
Each node, consisting of data and a reference to the next node, establishes a chain-like structure, enabling seamless insertion and deletion operations. This arrangement allows linked lists to offer superior memory allocation and dynamic sizing flexibilitycompared to arrays.
The non-contiguous data storage facilitated by nodes’ dynamic allocation in the memory enhances the ability to store and manage data effectively. Ultimately, the proficient use of nodes in linked lists underpins implementing more sophisticated data structures such as stacks and queues.
Advantages of Linked Lists
Having highlighted the role of nodes in linked lists, it becomes apparent that these lists’ advantages extend beyond efficient node utilization. They offer not only superior insertion and deletion operations compared to arrays but also facilitate dynamic memory management, easy implementation of other data structures, and flexible element updates.
- Efficient Insertion and Deletion: Linked lists, unlike arrays, allow for swift insertion and deletion of nodes at any point, without the need to shift other elements.
- Dynamic Memory Management: They enable dynamic allocation and deallocation of memory, eliminating the need for contiguous memory space.
- Implementation of Other Data Structures: Linked lists simplify the implementation of dynamic data structures like stacks and queues.
- Flexibility in Updates: They permit flexible updates and rearrangement of elements, enhancing their adaptability to dynamic data requirements.
Queue Data Structure Explained
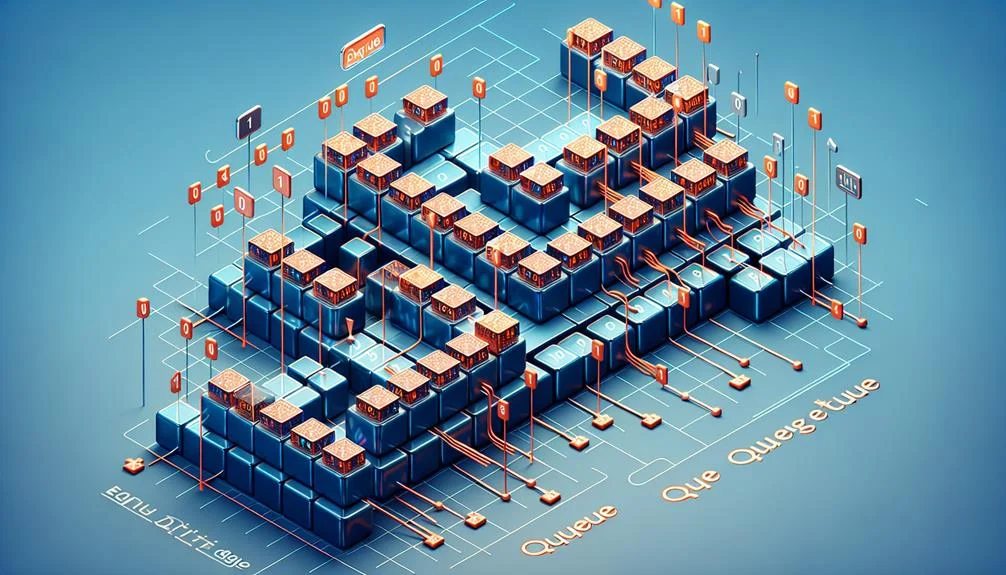
Queue data structures are linear structures that follow the FIFO (First In, First Out) principle, with elements inserted at the rear and removed from the front. This specific structure is employed in various scenarios, such as process scheduling, breadth-first search, and printer spooling, representing a fundamental component in computer science.
Two primary operations are linked with queues: enqueue and dequeue. Enqueue involves the addition of elements at the rear of the queue. Conversely, dequeue relates to the extraction of elements from the front, maintaining the FIFO principle, which guarantees that the first element added will be the first to be removed.
A variation of queues is circular queues. In these, the rear can loop around to the front, thus optimizing memory usage. This distinctive characteristic enhances the efficiency of queues, eliminating the wasted storage that occurs in linear queues. This continual movement—similar to a circle—ensures that every position can be utilized, making circular queues a significant asset in memory-conscious programming.
Deep Dive Into Binary Trees

In the hierarchy of complex data structures, binary trees stand out as an essential element. Each node has a maximum of two children nodes, rendering it a pivotal tool in efficient data management and search mechanisms. As hierarchical data structures, binary trees comprise root, internal, and leaf nodes, each playing a distinctive role in data management.
- The root node serves as the starting point, embodying the hierarchical nature of binary trees.
- Internal nodes, which connect other nodes, act as pathways, facilitating traversal methods such as in-order, pre-order, and post-order.
- Leaf nodes, having no children, signify the termination points in the tree.
- Binary search trees, a special type of binary tree, offer enhanced efficiency in search operations. They maintain a specific rule where each left child node has a lesser value than the parent node, and each right child node has a higher value.
Binary trees can be balanced or unbalanced, thereby affecting the complexities of search and insert operations. This careful consideration of the structure and properties of binary trees can vastly improve data handling efficiency in complex systems.
The Intricacies of Heaps
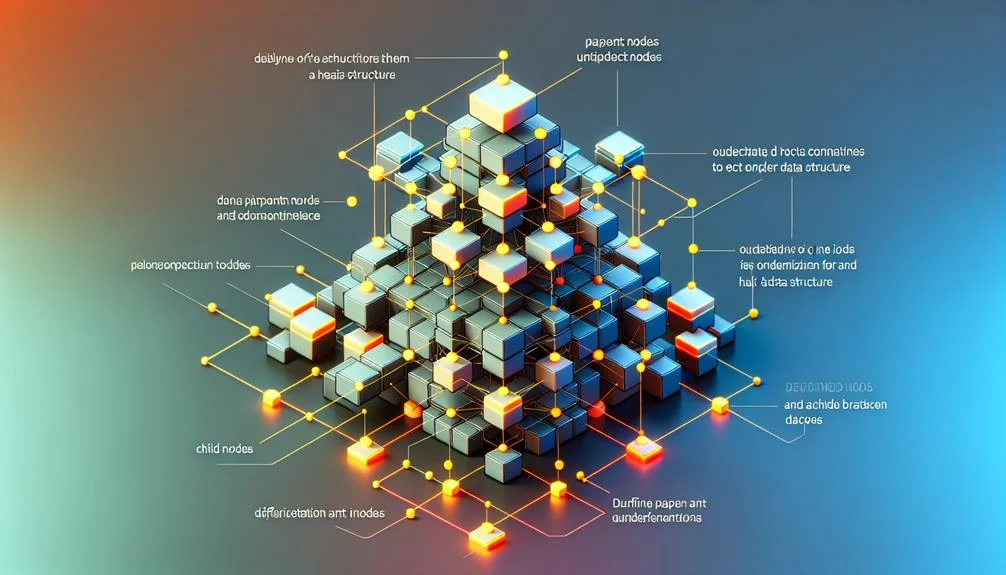
Moving forward in our exploration of data structures, we turn our attention to heaps, a specialized form of binary trees that guarantee a unique property, making them crucial in certain data manipulation operations like priority queues and heap sort algorithms.
Heaps are binary trees that adhere to the heap property, a specific structural condition that distinguishes them from other binary trees. There are two main types of heaps: max heaps and min heaps. In max heaps, the parent node is always greater than its children. Conversely, in min heaps, the parent node is consistently smaller than its children. This property ensures that the highest (max heap) or lowest (min-heap) priority element is always at the tree’s root.
This structural organization of heaps makes them highly efficient in certain operations, particularly in priority queues and heap sort algorithms. Priority queues benefit from the heap property, as elements with higher priority are served first. On the other hand, heap sort algorithms exploit this feature to sort elements in ascending or descending order. Moreover, heaps always form a complete binary tree, with the left child filled before moving to the right, ensuring efficient use of space.
Implementing Hashing Data Structures
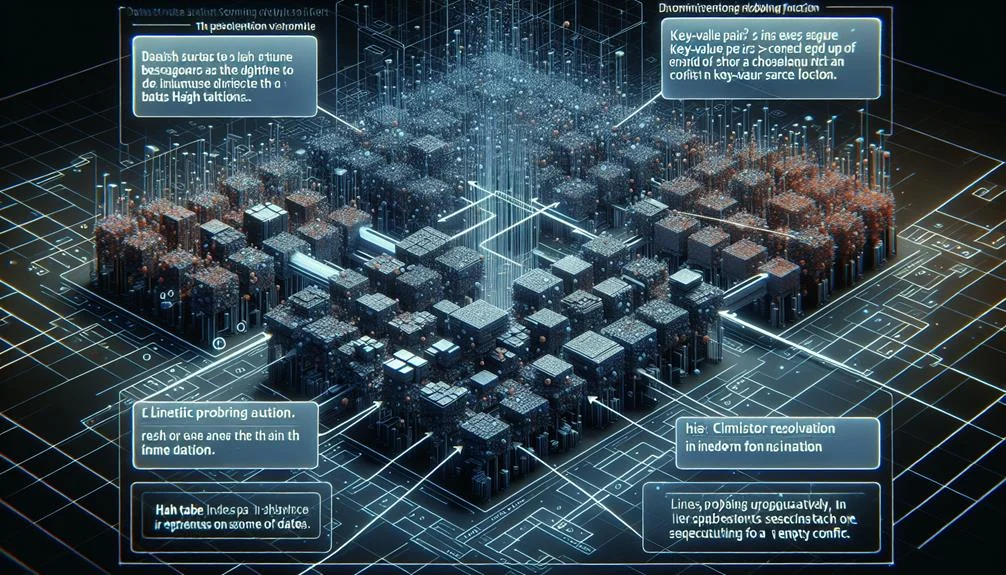
Understanding Hash Functions
To fully comprehend the implementation of hashing data structures, one must first grasp the concept and functionality of hash functions, which play an essential role in mapping data values to specific locations for swift and efficient access.
These functions generate a unique hash code, also known as a hash value, for each input. This code provides a direct pathway to the data’s location within the structure, enabling fast retrieval. However, collisions can occur when two different inputs produce the same hash value.
Understanding hash functions requires a detailed examination of their key components:
- The hash function itself, which generates the hash code.
- The hash value, which is the output of the hash function.
- The potential for collisions, when multiple inputs result in the same hash value.
- The need for collision resolution techniques, to address these conflicts and maintain data integrity within the hashing data structure.
Collision Resolution Techniques
Collision resolution technology rectifies the issue of multiple keys hashing to the same index. These techniques are fundamental to optimising hashing performanceg in data structure implementations.
Consider the following comparative analysis:
Technique | Description |
---|---|
Chaining | Linked list at each index to store colliding keys. |
Linear Probing | Searches for the next available slot in the hash table |
Quadratic Probing | Searches at quadratically increasing distances |
Double Hashing | Applies a second hash function when collision occurs |
Each technique offers unique trade-offs. Chaining facilitates the deletion of keys but can lead to poor cache performance. Linear probing provides better cache performance but suffers from clustering. Understanding these nuances enables the effective implementation of hashing data structures.
Conclusion
In summary, exploring data structures elucidates the profound intricacy of organizing and storing data. From the simplicity of arrays to the complex organization of heaps, each structure holds a unique place in data management.
Their implementation, while seemingly paradoxical in their complexity and simplicity, is crucial to efficient data handling. Undeniably, these mechanisms form the backbone of data-driven decision making, underscoring the ironic truth that the power of data lies not just in its volume, but in its structure.