Stacks in data structures refer to abstract data types that store or arrange data in a linear structure following the Last-In-First-Out (LIFO) principle. Essential operations include push (insertion), pop (removal), and peek (viewing top element). These operations primarily occur at the stack’s top. The stack can be implemented using arrays or linked lists and manipulates data efficiently. Understanding advanced stack operations including push, pop, peek, isFull, and isEmpty functions can greatly enhance stack potential.
- Stacks are data structures that follow the Last In First Out (LIFO) principle, with operations occurring at the top.
- Basic stack operations include insert (push), remove (pop), peek, isFull, and isEmpty functions for managing data.
- Stacks can be implemented using arrays or linked lists, allowing efficient data manipulation through push and pop operations.
- Time complexity of push and pop operations is O(1), highlighting the efficiency and scalability of stacks.
- Stacks are used in various applications such as function calls, recursion, undo functionalities, and expression evaluation.
Understanding Stacks in Data Structures
To fully grasp the concept of stacks in data structures, it is crucial to understand its representation, which follows the Last In, First Out (LIFO) principle, a distinct characteristic that defines its operational behaviour. This LIFO principle in a stack data structure indicates that the last element added to the stack is the first to be removed.
Stacks are linear data structures with a top and a base. The top of the stack is where all operations occur. This is because insertion (push) and deletion (pop), the key operations on a stack, happen at the top. The initial element added to the stack is at the base, and it is the last to be removed, maintaining the LIFO concept.
Stacks in data structures can be implemented using arrays or linked lists. Irrespective of the implementation method, the operational behaviour remains consistent. For example, when utilizing a linked list, the new element becomes the top element. This element is the first to be removed in subsequent operations. Fundamentally, the stack’s representation embodies its LIFO nature, vital to its data-handling functionality.
Basic Stacks in Data Structures Operations
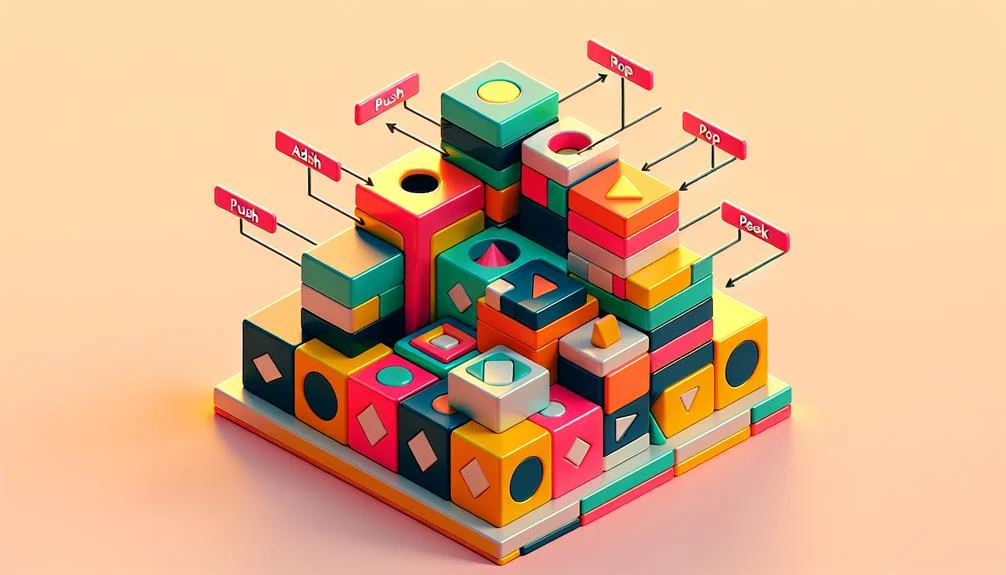
In stack data structures, fundamental operations such as insert and remove constitute the core of the Last In, First Out (LIFO) principle.
The insert operation is responsible for adding elements into the stack while the remove operation eliminates elements, following the LIFO concept.
Understanding and effectively implementing these operations is crucial for managing data within the stack structure and mitigating issues like stack underflow and overflow.
Understanding Stacks in Data Structures Operations
Stack operations include push and pop functions, which adhere to the Last In, First Out (LIFO) principle, fundamentally allowing the insertion and removal of elements from the top of the stack. This linear data structure embodies the LIFO methodology to guarantee efficient data manipulation.
Here are some of the basic operations:
- Push: Adds an element to the top of the stack.
- Pop: Removes an element from the top of the stack.
- Peek: Retrieves the topmost element without eliminating it from the stack.
- isFull & isEmpty: These functions check whether the stack is full or empty, respectively.
Understanding these operations is critical for effective data processing in diverse applications.
Implementing Push and Pop
Let’s explore the actual implementation of push and pop operations, fundamental tools in managing stack data structures with adherence to the Last In First Out (LIFO) principle.
In a push operation, an element is added to the top of the stack, extending its size to accommodate the new item in the data structure.
Conversely, the pop operation removes an element from the stack’s top, effectively reducing its size. These operations are essential for manipulating data efficiently within a stack.
Implementing Stacks in Data Structures Programming
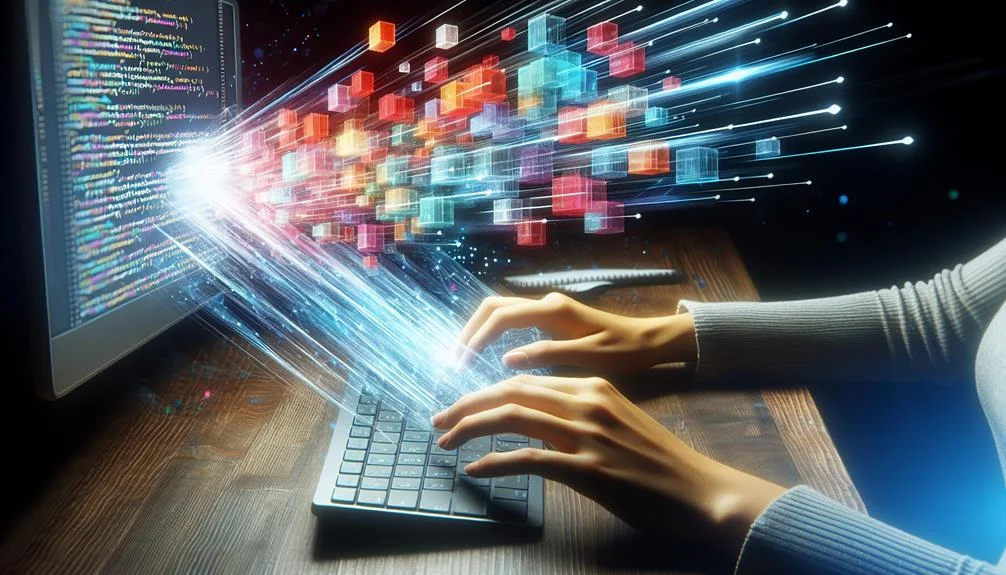
The process of implementing stacks in programming can be achieved through the use of either arrays or linked lists, each offering its unique approach for tracking the top element and performing operations.
This implementation allows for the efficient execution of fundamental stack operations such as push and pop. It is possible in various programming languages, including Python, Java, C, and C++, each necessitating the definition of stack-specific functionalities.
Stacks in Data Structures Operations Explanation
Understanding stack operations in programming requires a grasp of key functions such as push and pop, which are vital in manipulating data following the Last In First Out (LIFO) principle.
When implementing stacks, it’s crucial to manage a ‘top’ pointer, which keeps track of the top element in the stack. The effectiveness of stack operations is often achieved with array-based implementations in programming languages like Python, Java, C, and C++.
Stack operations include:
- Push: This operation increments the top pointer and adds a new element to the stack.
- Pop: This operation decrements the top pointer and removes the top element from the stack.
- isEmpty: This is used to check if the stack is empty before performing push or pop operations.
- isFull: This checks if the stack is full prior to performing push or pop.
These operations ensure efficient data manipulation in stack structures.
Real-world Applications of Stacks in Data Structures
In programming, stacks play an indispensable role in managing function calls and recursion, offering efficient undo mechanisms for user actions, facilitating expression evaluation using postfix notation in compilers and calculators, powering backtracking algorithms to explore possible solutions, and even enabling browser history functionality.
Implementing stacks in various real-world applications is a confirmation of their versatility and efficiency. To illustrate their usage, let’s look at a table that shows some typical applications of stacks:
Application | Description | Stack Utilization |
---|---|---|
Function Calls | Manages sequence of operations | Handles function call and return |
Undo Mechanism | Reverts user actions | Stores the previous state |
Expression Evaluation | Calculates arithmetic expressions | Helps in translating and evaluating expressions |
Browser History | Tracks web pages visited | Last visited page is always on top |
In essence, stacks are not just practical tools in solving complex programming tasks but also serve as a validation of their effectiveness.
Stacks in Data Structures Time Complexity
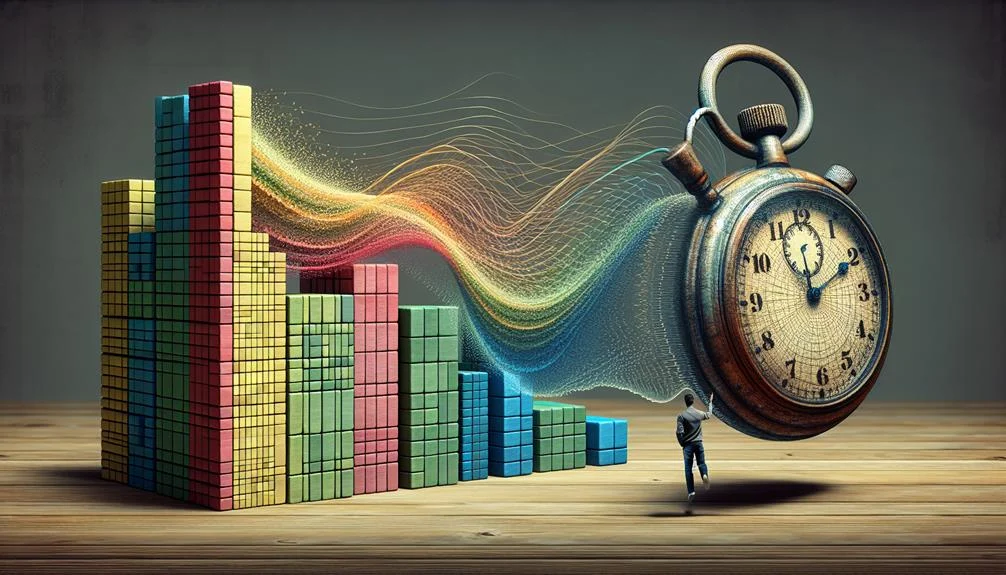
Looking into the concept of time complexity in stacks, we find that for array-based implementations, the push and pop operations exhibit a constant time complexity of O(1), irrespective of the number of elements in the stack. This characteristic of stacks is fundamental to their efficiency, particularly in basic operations such as adding (push) and removing (pop) items.
To understand this concept better, consider these points:
- Time complexity O(1) implies that the time taken to perform either a push or a pop operation remains constant, independent of the stack’s size.
- The efficiency of stacks is underscored by this constant time complexity, making them suitable for scenarios where rapid addition and removal of items are required.
- The time complexity remains unaltered even as the number of elements in the stack increases, illustrating the scalability of stack implementations.
- Evaluating time complexity is vital when evaluating the performance of different stack implementations in various scenarios.
The O(1) time complexity of push and pop operations in stacks underscores their functionality and efficiency in handling large data sets.
Application of Stacks in Data Structures
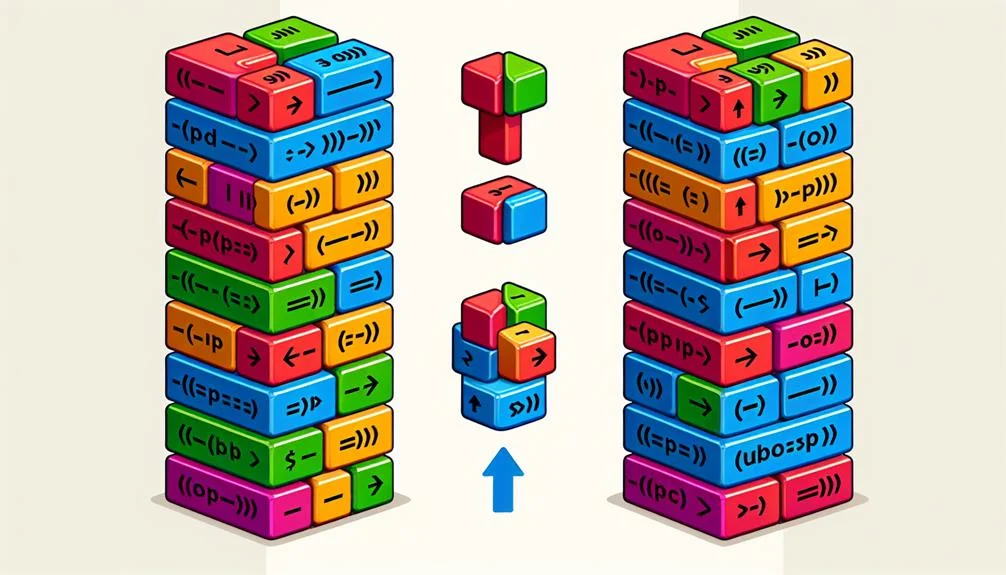
From managing function calls in programming to enabling ‘undo’ operations in software, stack data structures serve many purposes in various computational contexts. In function calls and recursion mechanisms, stacks are pivotal. They systematically manage function calls and their respective return points, ensuring smooth changes between nested and parent functions. This is essential in recursion, where functions call themselves multiple times.
Stacks also play a critical role in software applications that offer ‘undo’ functionalities. They track the sequence of actions performed and allow operations to be reversed in a last-in, first-out (LIFO) manner. This mechanism also applies in managing browser history, where the most recent web pages are accessed first.
Expression evaluation, particularly in postfix notation, leverages stacks for efficient computation. Similarly, backtracking algorithms, which explore possible solutions before reverting to a previous step, utilize stacks for memory management.
Here is a table summarizing some applications of stack data structures:
Application | Description | Keyword |
---|---|---|
Function Calls & Recursion | Managing function calls and return points | Memory Management |
Undo Functionalities | Reverses operations in LIFO manner | Backtracking Algorithms |
Expression Evaluation | Helps in computation of postfix notation | Postfix Notation |
Stacks in Data Structures Case Studies
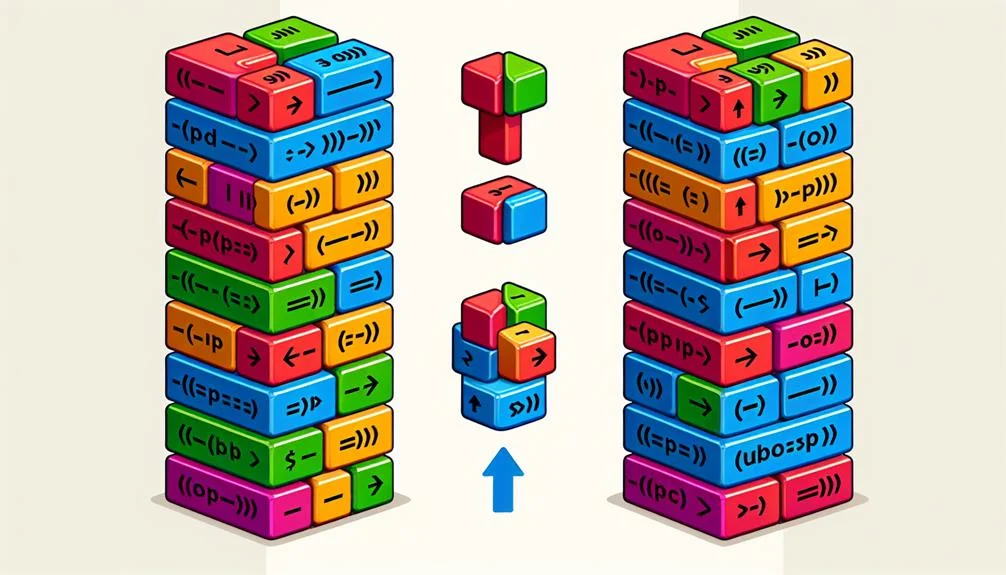
Stack data structures are often implemented using arrays or linked lists, each offering unique advantages in terms of efficiency and versatility, which is crucial for various computational needs and use cases.
Case studies have shown that Array Implementation can offer efficient push-and-pop operations. This is because arrays provide direct access to any index, which makes these operations fast and consistent.
On the other hand, Linked List Implementation offers a more flexible approach. Linked lists allow for easy addition and removal of elements from the stack, which can be advantageous in situations where the stack size might change dynamically.
Speaking of dynamic changes, Dynamic Array Usage is an effective way to handle variable stack sizes. Dynamic arrays can grow or shrink as required, providing a flexible solution for stack implementation.
Finally, both array and linked list implementations allow for Efficient Space Utilization. This makes stacks a Fundamental Data Structure in various computational paradigms, as they provide efficient memory usage.
- Array Implementation: Fast push and pop operations.
- Linked List Implementation: Flexibility with dynamic stack sizes.
- Dynamic Array Usage: Efficient handling of variable stack sizes.
- Efficient Space Utilization: Optimal use of memory.
Advanced Concepts of Stacks in Data Structures
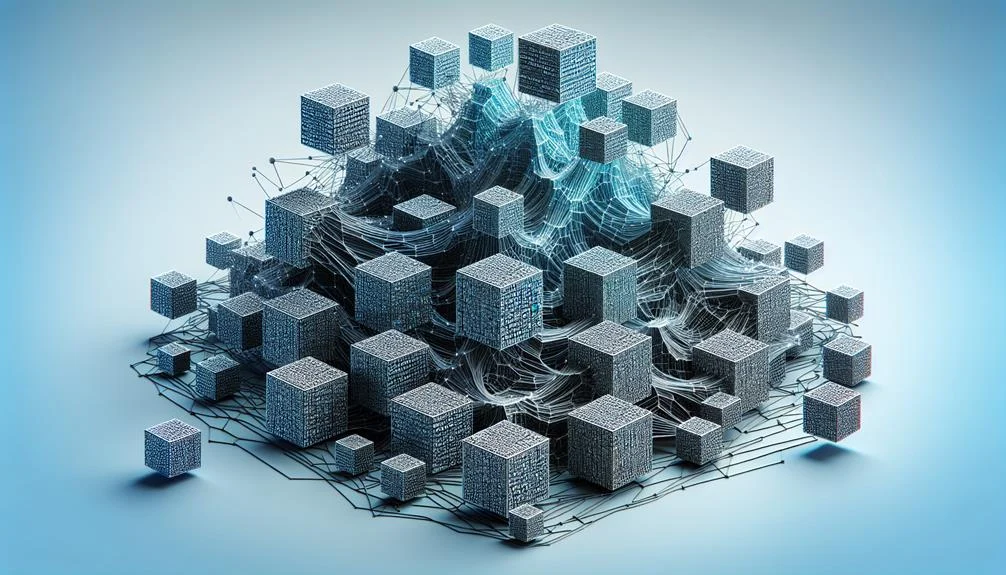
Implementations of stack structures can be executed proficiently using arrays or linked lists, with advanced implementations introducing additional operations such as peek, isFull, and isEmpty.
The peek operation allows us to view the top element of the stack without removing it, thereby preserving its structure. The isFull and isEmpty operations are essential for managing stack capacity and preventing overflow or underflow errors.
To optimize the efficiency of stack operations, specialized stack designs have been developed that allow the retrieval of minimum values in O(1) time and space. This optimization is a significant advancement in the domain of data structures.
Stacks can be simulated using priority queues or heaps, broadening their application in various domains. This simulated structure can significantly impact algorithm design and streamline problem-solving processes. Understanding these advanced concepts is essential for leveraging the full potential of stack data structures.
Conclusion
Stacks in data structures play a pivotal role in computer science. They enable the efficient execution of both simple and complex algorithms. Their LIFO principle, ease of implementation, and versatility make them invaluable for myriad applications, from parsing expressions to managing function calls.
Understanding their intricacies, including their operations, time complexity, and potential use cases, is fundamental for any aspiring or practising software engineer. Continuing advancements in stack data structures promise more optimized and innovative solutions for future computational challenges.