Linked lists are a type of dynamic data structure composed of elements, or nodes, each containing data and a reference to the next node in the sequence. Different types include singly, doubly, circular, multi-level, and self-referential linked lists. They allow efficient insertion and deletion operations without shifting elements, making them an advantageous choice for managing dynamic data. Nodes are interconnected for easy data traversal, with certain types offering bidirectional traversal functionality. As essential building blocks in computer science, linked lists are integral to numerous applications, from browser history management to algorithm optimization.
Main Points
- Linked lists are dynamic data structures composed of nodes with data and pointers for interconnection.
- They permit efficient operations such as traversal, insertion, deletion, and searching without shifting elements.
- Types of linked lists include singly, doubly, circular, multi-level, and self-referential, each with unique pointer arrangements.
- Nodes in a linked list are dynamically allocated and interconnected through pointers, allowing fluid data management.
- Applications of linked lists include web browsing history, music playlists, text editor functionalities, graph algorithms, and operating system processes.
Understanding Linked Lists
Linked lists in data structures, are collections of nodes, each containing data and a pointer to the succeeding node. As a dynamic data structure, linked lists allocate memory as needed, making them a versatile tool for programmers. Their dynamic memory feature inherently supports the efficient execution of operations such as insertion and deletion without requiring the shift of elements, unlike arrays.
Nodes within a linked list are interconnected through pointers, facilitating easy traversal of data elements. The nodes, which consist of data and a ‘next’ pointer, form the backbone of the list. The ‘next’ pointer provides the linkage to the subsequent node, thereby creating the chain-like structure of a linked list.
In a doubly linked list, an additional pointer is present in each node to reference the preceding node, permitting bidirectional traversal. This enhances the flexibility and functionality of linked lists.
Linked lists are fundamental linear data structures that provide an efficient mechanism for data management in dynamic programming scenarios. They play an important role in various algorithms and applications, primarily due to their dynamic memory allocation, efficient data insertion, and deletion capabilities.
Types of Linked Lists
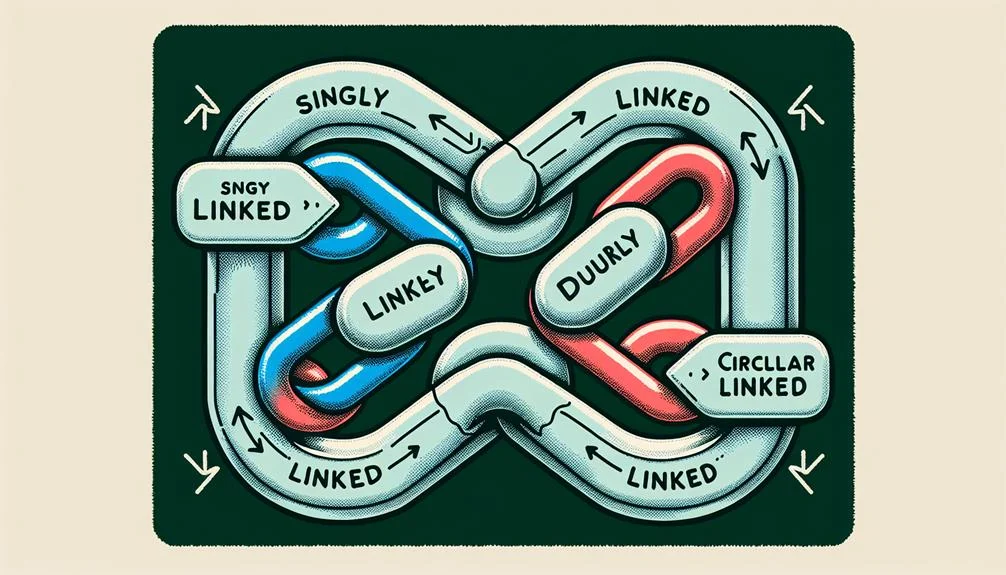
The singly linked list is the most basic type, where each node only carries a reference to the next node, facilitating linear traversal. In contrast, the doubly linked list allows bidirectional traversal, as nodes contain pointers to both the next and previous nodes.
The circular linked list is unique in its structure, the final node loops back to reference the first, creating a circular connection. In the multi-level linked list, nodes can point to multiple levels of nodes, adding complexity. Finally, the self-referential linked list has nodes that can refer to themselves, creating intriguing self-referential structures.
Here is a concise summary of these linked list types:
TYPE | KEY ATTRIBUTE | TRAVERSAL TYPE |
---|---|---|
Singly | Reference to next node | Linear |
Doubly | Next & previous nodes | Bidirectional |
Circular | Final node to first node | Circular |
Multi-level | Multiple level references | Multi-Level |
Self-referential | Node refers to itself | Self-referential |
This overview should provide a solid foundation for further exploration of linked lists in data structures.
Operations in Linked Lists
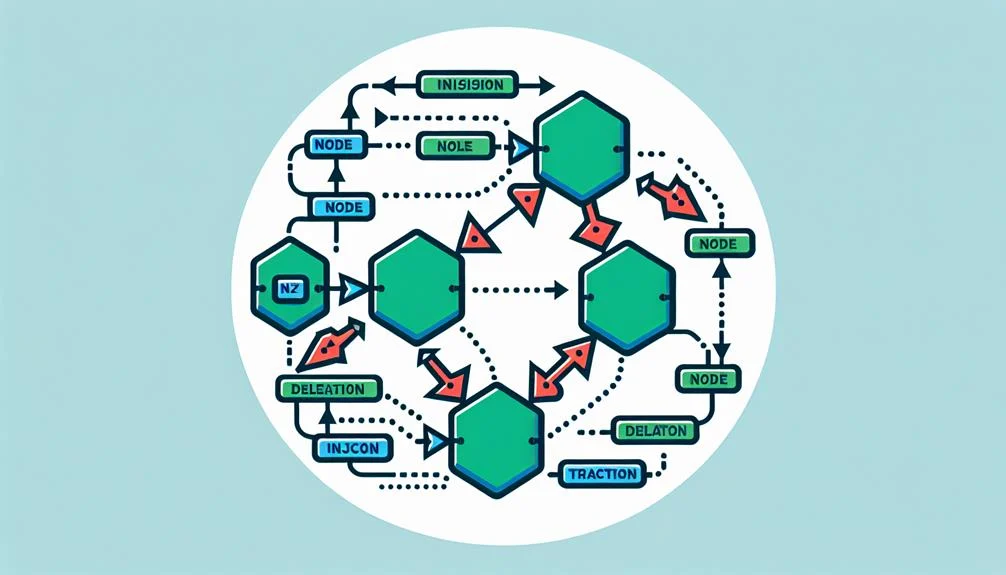
After gaining an understanding of the various types of linked lists, we can now shift our attention to the operations that can be performed on these data structures, starting with the fundamental operations such as traversal, insertion, deletion, searching, and reversing.
Traversal is an essential operation that involves visiting each node in the linked list sequentially. This operation is important in computer science as it facilitates the examination of all the nodes in the linked list.
The insertion operation allows for the addition of nodes at the start, middle, or end of the list, thereby providing flexibility in managing data within the list.
The deletion operation in linked lists removes nodes from any position in the list. It is an important operation when it comes to memory management and efficient data handling.
Searching within a linked list is another operation that locates a specific node based on its value, providing a means to organize and retrieve data.
The reversing operation modifies the linked list such that all the links to the nodes are reversed, enabling backward traversal of the list. Understanding these operations enhances one’s grasp of data structures and contributes to more efficient programming.
Linked List Implementation
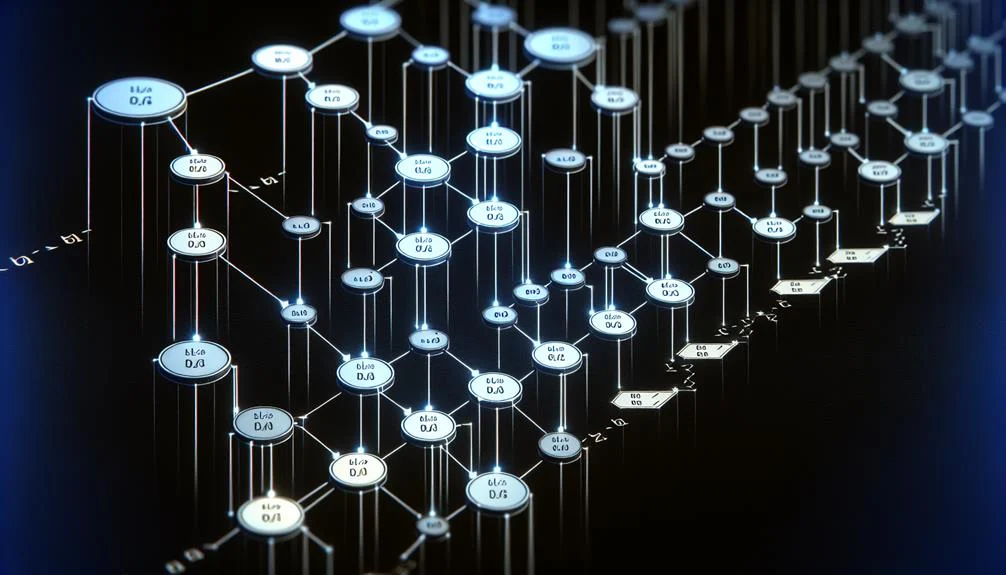
Implementing a linked list involves creating nodes that not only house data elements but also contain a reference to the next node in the structure, ensuring a fluid connection between data points. The implementation of linked list data structures is centered around these nodes, each having two components: the data itself and the “next pointer”. This pointer holds the memory address of the subsequent node, forming the link between nodes.
Nodes are dynamically allocated in memory, providing flexibility in list size adjustments. This dynamic data allocation allows for efficient memory usage, a key benefit of linked list implementation.
Operations such as insertion and deletion in a linked list are straightforward. For insertion, a new node is created and its address is updated in the previous node’s next pointer. Deletion involves removing the specific node’s address from the previous node’s next pointer, effectively excluding it from the list.
Here is a table summarizing the key aspects:
Aspect | Description |
---|---|
Node | Houses data and reference to next node |
Dynamic Allocation | Nodes are dynamically allocated in memory |
Insertion | New node is created and its address is updated in previous node’s next pointer |
Deletion | Specific node’s address is removed from previous node’s next pointer |
Through careful management of nodes, addresses, and pointers, linked lists offer a dynamic and efficient data structure.
Applications of Linked Lists
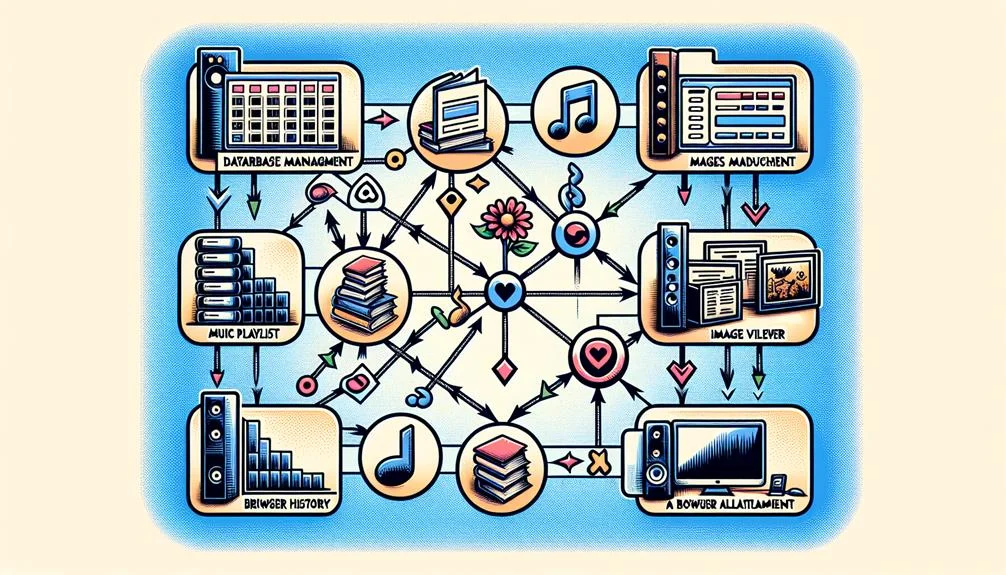
Building on the foundational understanding of linked list implementation, we now turn our attention to the diverse applications of linked lists in various domains, from web browsers and music player applications to text editors, graph algorithms, and operating systems.
Web browsers use linked lists to manage browser history and tabs. Each node represents a webpage, facilitating efficient webpage navigation. Similarly, music applications harness linked lists to create playlists, where each node represents a song, ensuring sequential play and easy insertion and deletion of songs.
In text editors, linked lists support the undo and redo functionalities. Each node stores a user action, making it possible to traverse back and forth the list to undo or redo actions. Graph algorithms, like Dijkstra’s, exploit linked lists to portray graph structures, optimizing path finding.
In operating systems, linked lists play a pivotal role in process scheduling, memory management, and file system organization. Each node in the linked list representation can represent a process, a memory block, or a file, respectively. Such organization bolsters system performance by ensuring efficient resource utilization and management.
Conclusion
In summary, the understanding and application of linked lists in data structures is essential in the field of computer science. They offer an efficient way to perform operations such as insertion, deletion, and traversal.
Their flexibility, ease of use, and dynamic size allocation make them a preferred choice in various applications, from implementing stacks and queues to managing memory in operating systems.
Therefore, mastering linked lists is an important step towards effective data management and algorithm development.