The binary search algorithm, a fundamental tool in computer science and data processing, stands as a method of strategic efficiency in locating specific values within sorted arrays. Its systematic approach of dividing and conquering the search space offers a glimpse into the intricacies of problem-solving within vast datasets. Understanding the nuances of this algorithm not only aids in precise target identification but also reveals a broader perspective on the strategic underpinnings of computational intelligence.
Key Takeaways
- Efficiently locates target value in sorted arrays.
- Follows divide and conquer strategy.
- Time complexity of O(log n).
- Ideal for larger datasets.
- Requires sorted array for effective operation.
Definition and Purpose of the Binary Search Algorithm
The binary search algorithm, with its systematic approach of dividing the search interval in half, efficiently locates a target value in a sorted array.
This algorithm operates by comparing the target value with the middle element of the array. If the middle element is not the target, the search space is divided in half, and the process is repeated in the appropriate subarray.
The primary purpose of the binary search algorithm is to quickly locate a specific element within the sorted array while greatly reducing the time complexity to O(log n) compared to linear search methods.
By following a divide and conquer strategy, the algorithm systematically narrows down the search space, making it particularly effective for large datasets.
Its objective is to swiftly determine the position of the target value through iterative or recursive comparisons, thereby enhancing search efficiency in sorted arrays.
Working Mechanism Explained
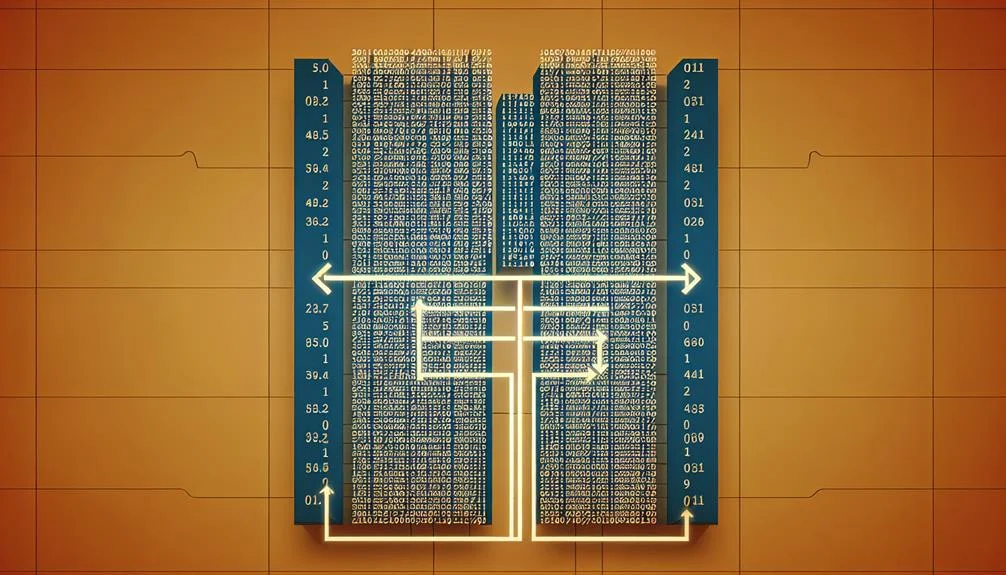
To explore the working mechanism of the binary search algorithm, it is important to understand how the iterative process of dividing the search interval in half plays a pivotal role in efficiently locating target values within a sorted array.
Initially, the algorithm identifies the middle element of the sorted array and compares it to the target value. Based on this comparison, it either concludes that the target value is equal to the middle element, lies to the left (smaller values), or to the right (larger values).
By applying the principle of ‘divide and conquer,’ binary search eliminates half of the remaining elements in each iteration, greatly reducing the search space. This process continues recursively on the remaining half until the target value is found or the interval becomes empty.
As a result, binary search is highly efficient for quickly locating elements in a sorted array by intelligently dividing the search space based on comparisons with the middle element.
Time Complexity Analysis
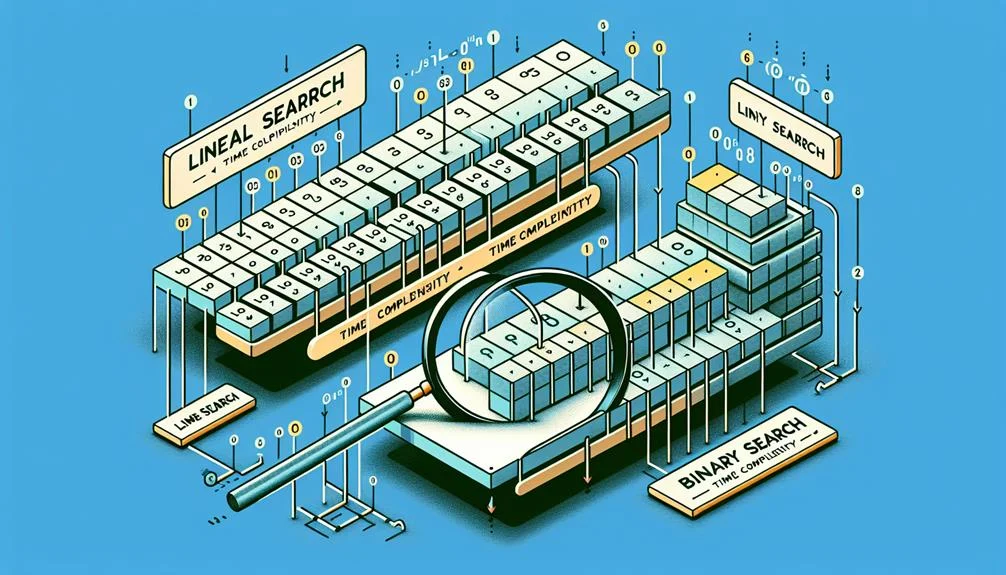
In analyzing the time complexity of the binary search algorithm, a key aspect worth examining is its efficient division of the search interval to locate target values within a sorted array. With a time complexity of O(log n), where n represents the number of elements in the array, binary search excels in efficiency by halving the search space at each iteration.
This logarithmic growth in the number of comparisons required showcases the algorithm’s ability to swiftly narrow down the potential locations of the target element. Compared to linear search, binary search is particularly advantageous for larger datasets due to its efficient nature.
Understanding the time complexity of binary search not only provides insights into its performance but also highlights the algorithm’s effectiveness in quickly identifying the desired element within a sorted collection. This analysis underscores the strategic approach employed by binary search in efficiently locating elements within arrays.
Necessary Conditions for Implementation
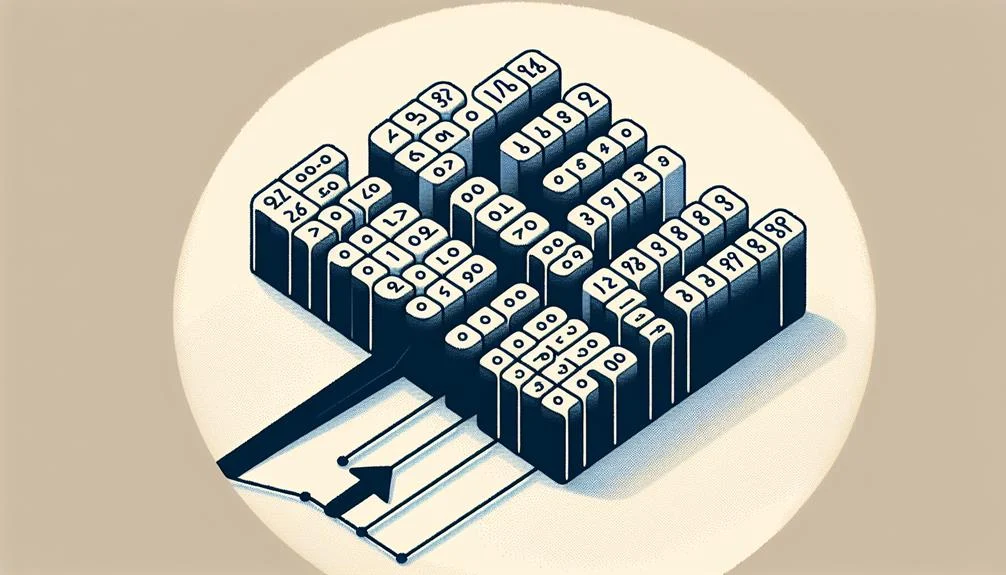
For successful implementation of the binary search algorithm, the array must be sorted in either ascending or descending order. The algorithm relies on the sorted nature of the array to efficiently locate a specific element by repeatedly dividing the search interval in half.
By ensuring the array is sorted, binary search can compare the target value with the middle element of the array and determine whether the target lies in the lower or upper half of the current interval. This process of halving the search space at each step allows binary search to quickly converge on the desired element, making it a highly efficient search algorithm.
The sorted order of the array is essential for the algorithm to function correctly and deliver the expected logarithmic time complexity of O(log n) when searching for elements in large datasets.
Handling Unsorted Arrays
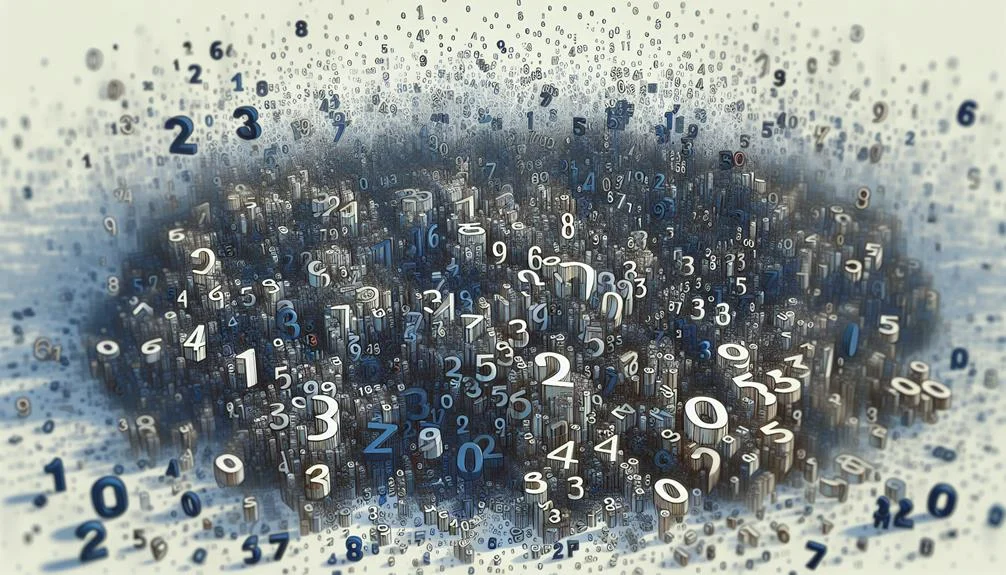
When dealing with unsorted arrays in the context of the binary search algorithm, the foremost step is to address the issue of sorting such arrays.
Sorting is imperative for binary search to function correctly, as the algorithm’s efficiency hinges on the ordered nature of the data.
Hence, the process involves sorting unsorted arrays, considering the time complexity implications, and then implementing binary search for accurate and reliable results.
Sorting Unsorted Arrays
Sorting unsorted arrays is a prerequisite step before applying the binary search algorithm effectively. Binary search relies on the array elements being in a sorted order to efficiently find the target value.
When dealing with unsorted arrays, sorting becomes crucial to guarantee the binary search can function correctly. Various sorting algorithms such as quicksort or mergesort can be utilized to rearrange the elements into a sorted sequence. These sorting algorithms provide efficient methods for finding the desired element within the array using binary search.
Time Complexity Considerations
Considering the necessity of sorting unsorted arrays for best performance in the binary search algorithm, time complexity considerations play a critical role in determining the efficiency of handling such data structures.
When dealing with unsorted arrays, the binary search algorithm’s typical time complexity of O(log n) can be notably impacted. This is because sorting the array prior to conducting the binary search introduces an extra time complexity that can make the overall process less efficient for unsorted data sets.
To address this challenge, various sorting algorithms like quicksort, mergesort, or heapsort can be employed to preprocess the unsorted array and bring it to a state where binary search can be effectively applied.
Implementing Binary Search
Efficiently implementing the binary search algorithm on unsorted arrays necessitates a preliminary step of sorting the data to facilitate accurate search operations. When dealing with unsorted arrays, the following steps are important for successful binary search implementation:
- Sorting the Array: Before applying the binary search algorithm, it is essential to sort the unsorted array using efficient sorting techniques such as quicksort or mergesort.
- Securing Accuracy: Sorting the array guarantees that the binary search algorithm can effectively locate the desired element with precision and reliability.
- Enhancing Efficiency: By sorting the array beforehand, the binary search operation becomes more efficient, as searching in a sorted array reduces the search space with each comparison.
- Managing Complexity: While sorting the array introduces an extra step, the benefits of accurate and efficient binary search results outweigh the added complexity of handling unsorted arrays.
Implementing binary search on unsorted arrays requires the initial step of sorting to transform the data into a format conducive to the algorithm’s efficient operation.
Application Beyond Numeric Data
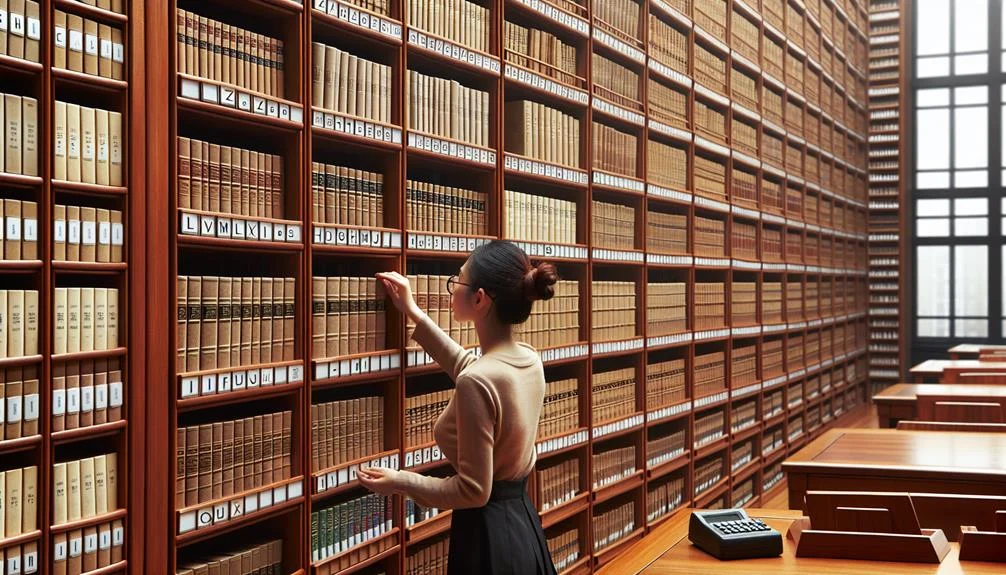
The application of the binary search algorithm extends beyond numeric data to include searching for specific values in arrays of strings, objects, or custom data types.
This algorithm’s efficiency in locating target values in sorted arrays is not constrained by the data type, allowing for fast and accurate searches in various data structures.
Whether searching for textual data, image data, or other non-numeric information, the binary search algorithm proves to be a versatile and valuable tool in diverse applications.
Non-Numeric Data Search
Binary search algorithm can effectively search for non-numeric data in sorted arrays by comparing elements based on their defined order or criteria. When dealing with non-numeric data, such as strings, characters, or custom objects, binary search remains a powerful tool for efficiently locating specific elements within a sorted collection.
To successfully apply binary search to non-numeric data, it is essential to have a clear ordering mechanism in place to determine how elements are compared and positioned within the array. By following the same principles of dividing the search space in half and strategically evaluating the elements, binary search can navigate through sorted arrays of non-numeric data with precision and speed.
- Binary search can be used with strings and characters in sorted arrays.
- Custom objects can be searched using binary search algorithm.
- Non-numeric data elements are compared based on a defined ordering mechanism.
- The algorithm efficiently locates non-numeric elements by dividing the search space in half.
Textual Data Search
When searching for textual data beyond numeric values, the binary search algorithm demonstrates its effectiveness by comparing target strings with the middle element in a sorted array. This method allows for efficient searching in alphabetical order, making it ideal for applications that require finding specific words or phrases in sorted text databases.
Textual binary search requires the data to be arranged alphabetically to guarantee accurate and rapid search results. This approach finds practical use in word dictionaries, spell checkers, and auto-complete features in text editors, enhancing the user experience by providing quick and precise search capabilities.
Advantages of Textual Binary Search | ||
---|---|---|
Efficient | Effective | Accurate |
Fast | Precise |
Image Data Search
In data search applications, the utilization of binary search algorithms extends beyond textual data into the field of image data search. Image data search involves using binary search algorithms efficiently to locate specific images within a collection based on attributes or features.
Here are key points regarding image data search with binary search:
- Features like color, texture, shape, or metadata are utilized to index and search for images efficiently using binary search.
- Binary search in image data retrieval enables quick and accurate identification of similar or relevant images in large databases.
- By effectively dividing the search space, binary search algorithms can narrow down image matches based on similarity metrics.
- Techniques like hashing, indexing, or machine learning algorithms can enhance image data search with binary search, leading to improved performance and accuracy.
Incorporating binary search into image data search processes can greatly contribute to the efficiency and effectiveness of image retrieval tasks.
Conclusion
To sum up, the binary search algorithm efficiently locates target values within sorted arrays through a divide and conquer strategy with a time complexity of O(log n).
Its systematic approach to narrowing down the search space makes it ideal for large datasets. By comparing the middle element to the target value, binary search quickly identifies the position of the target element.
Its effectiveness in searching for data highlights its importance in algorithmic problem-solving.