The Bubble Sort algorithm, a fundamental sorting technique, operates by systematically comparing adjacent elements within a list and rearranging them if needed. This straightforward method initiates with the first two elements, ensuring they are placed in the correct order. Through subsequent passes, larger elements gradually shift toward their appropriate positions, creating a sorted sequence. The unique ‘bubbling’ mechanism of this algorithm intrigues many with its step-by-step approach to sorting arrays. Understanding the intricate details behind this seemingly simple process sheds light on the efficiency and nuances of Bubble Sort that make it a notable algorithm in sorting techniques.
Key Takeaways
- Bubble Sort iterates through the list, comparing and swapping adjacent elements.
- It gradually moves the largest unsorted element to its correct position.
- Elements ‘bubble’ up through passes, shifting towards ascending order.
- Swapping adjacent elements facilitates the repositioning process.
- Bubble Sort has a time complexity of O(n^2) and space complexity of O(1).
Bubble Sort Overview
The Bubble Sort algorithm, a fundamental sorting technique, compares adjacent elements and performs swaps to arrange them in the correct order. This sorting method works by iterating through the list of elements, comparing each pair of adjacent elements, and swapping them if they are in the wrong order.
The algorithm continues this process until the entire list is sorted in ascending order. The visualization of the algorithm involves elements gradually ‘bubbling’ or moving to their correct positions as the sorting progresses. Bubble sort is well-known for its simple implementation, making it a popular choice for educational purposes to understand the basics of sorting algorithms.
Despite its ease of implementation, the Bubble Sort algorithm has a time complexity of O(n^2), where ‘n’ represents the number of elements in the list, making it less efficient compared to other more advanced sorting algorithms.
Basic Steps of Bubble Sort
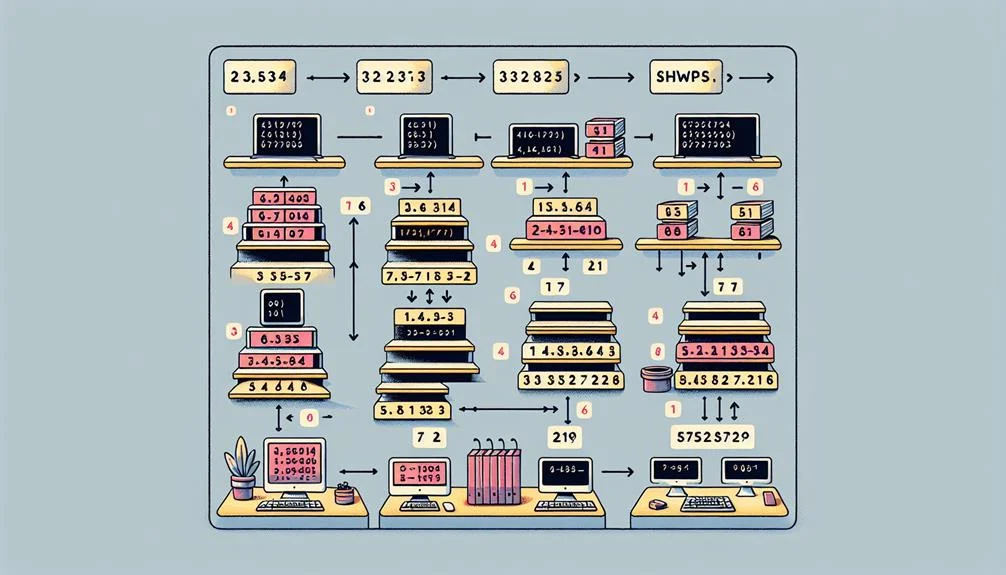
Execution of the Bubble Sort algorithm involves iteratively comparing and swapping adjacent elements to gradually arrange them in the correct order.
To implement Bubble Sort, the algorithm starts by comparing the first two elements in the list, swapping them if they are in the wrong order. It then moves to the next pair of elements and continues this process throughout the list.
This comparison-based sorting algorithm operates with a time complexity of O(n^2), where ‘n’ represents the number of elements in the list. The space complexity of Bubble Sort is O(1) as it only requires a constant amount of extra space.
Bubble Sort’s simplicity and ease of implementation make it a suitable choice for small datasets or educational purposes. However, due to its quadratic time complexity, it is not efficient for sorting large arrays, and other sorting algorithms like Quick Sort or Merge Sort are preferred for larger datasets.
Swapping Mechanism in Bubble Sort
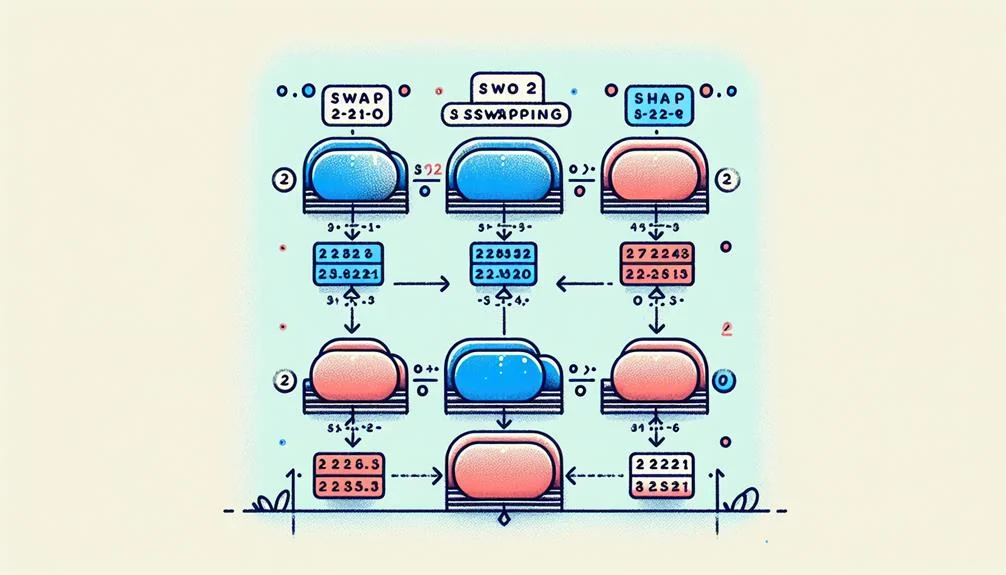
During the sorting process in Bubble Sort, elements are rearranged through a swapping mechanism based on their comparisons.
The swapping mechanism in the Bubble Sort algorithm functions by comparing adjacent elements in the array. If the current element is larger than the next element, a swap occurs, moving the larger element to the right. This swapping process guarantees that larger elements gradually shift towards their correct positions in the array.
Bubble sort is a comparison-based sorting algorithm, and the swapping mechanism plays a critical role in arranging elements in ascending order. The algorithm iterates through the array multiple times, each time pushing the larger elements towards their appropriate places.
Understanding the swapping mechanism is fundamental to comprehending how Bubble Sort effectively reorders elements for sorting purposes. Essentially, the swapping process is the key action that enables Bubble Sort to organize elements in the desired order efficiently.
Iterative Passes in Bubble Sort
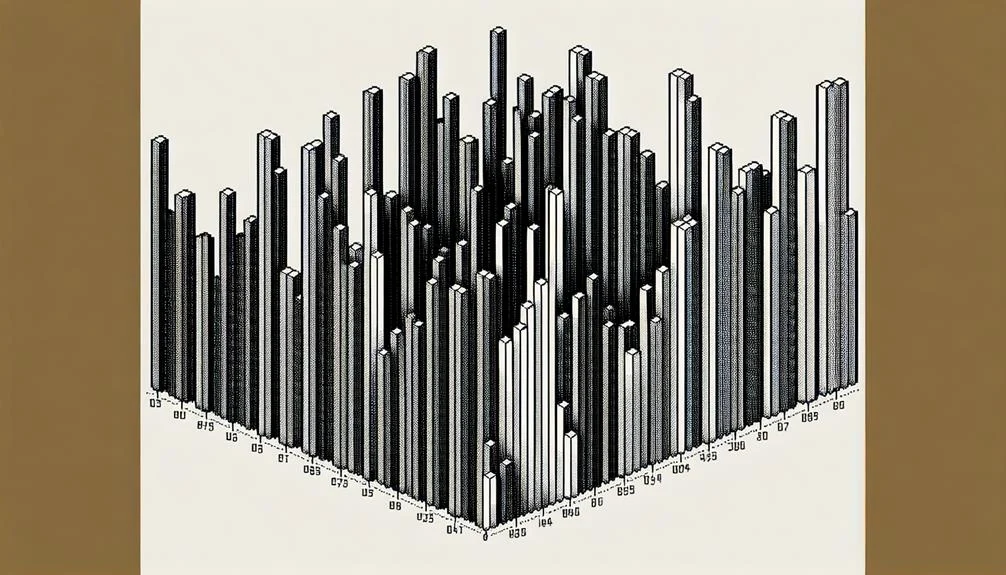
In Bubble Sort, iterative passes play a crucial role in gradually sorting the elements of an array. The algorithm works by comparing adjacent elements in the array and swapping them if they are in the wrong order. During each pass, the largest unsorted element is moved to its correct position in the array.
This process of moving the largest unsorted element continues with multiple passes until no further swaps are required, indicating that the list is fully sorted. Visually, Bubble Sort resembles elements ‘bubbling’ to their correct positions as the algorithm progresses.
The number of passes needed for sorting varies depending on the initial order of elements and the total number of elements in the list. Through these iterative passes, Bubble Sort efficiently rearranges the elements until every element finds its rightful place, resulting in a sorted array.
Movement of Elements in Bubble Sort
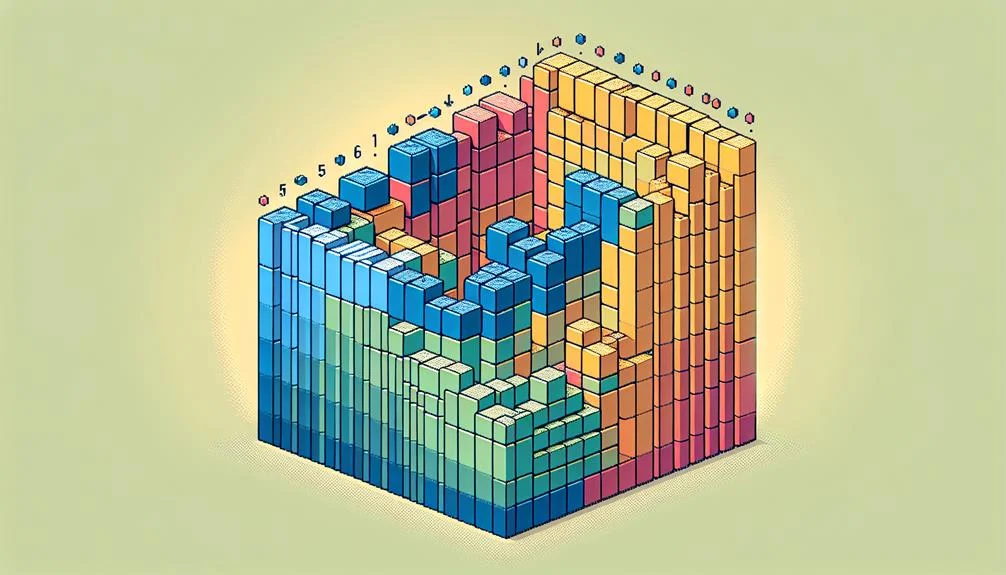
The movement of elements in the Bubble Sort algorithm involves the systematic swapping of adjacent elements during each pass. Through repeated passes, the larger elements gradually bubble up to their correct positions within the array.
This process continues until all elements are sorted in ascending order, showcasing the iterative nature of Bubble Sort’s element movement.
Swapping Adjacent Elements
The swapping of adjacent elements in the Bubble Sort algorithm facilitates the gradual repositioning of elements within the array. This swapping process is initiated by comparing neighboring elements in the array and exchanging them if they are out of order.
As the algorithm progresses through iterative passes, the larger elements are continuously shifted towards the end of the array in ascending order. The mechanics of swapping adjacent elements in Bubble Sort play a vital role in the sorting algorithm’s operation, enabling the systematic rearrangement of elements until the entire array is properly sorted.
Understanding the intricacies of how elements move during these swaps is essential for comprehending the iterative nature and progression of the Bubble Sort algorithm. Through the careful manipulation of adjacent elements, Bubble Sort effectively organizes the array into the desired ascending order, showcasing the algorithm’s step-by-step approach to sorting.
Repeated Passes Through
During the repeated passes through the array in the Bubble Sort algorithm, elements gradually shift towards their correct positions through iterative comparisons and swaps.
This comparison-based sorting technique operates by examining adjacent elements and rearranging them if they are not in the desired order. As the algorithm progresses through each pass, larger elements move up the array by swapping with smaller adjacent ones until they reach their appropriate place in the ascending order.
The iterative nature of Bubble Sort guarantees that each element progresses towards its correct position through multiple comparisons and exchanges with neighboring elements. This repetitive process of moving elements closer to their sorted locations continues until the entire array is arranged in the correct order.
The term ‘bubble’ in Bubble Sort reflects how elements rise or bubble up in the array as they gradually shift to their rightful positions with each iteration.
Element Bubbling to Place
Element movement in Bubble Sort is a fundamental aspect that drives the gradual sorting process in the algorithm. Elements progressively shift towards their correct positions through iterative comparisons and swaps.
In the Bubble Sort algorithm, elements in an array are compared pairwise. If they are out of order (not in ascending order), a swap is performed to reposition them correctly. As the algorithm progresses through multiple passes, the largest unsorted element in the array gradually moves to its sorted position with each comparison and swap.
This movement of elements mimics bubbles rising to the surface, inspiring the name ‘Bubble Sort’. The process of element bubbling to place continues until all elements are in their correct sorted order.
Through this repetitive comparison and swapping process, Bubble Sort efficiently reorganizes the elements in ascending order. This makes it a straightforward yet effective sorting algorithm.
Sorting Sequence in Bubble Sort
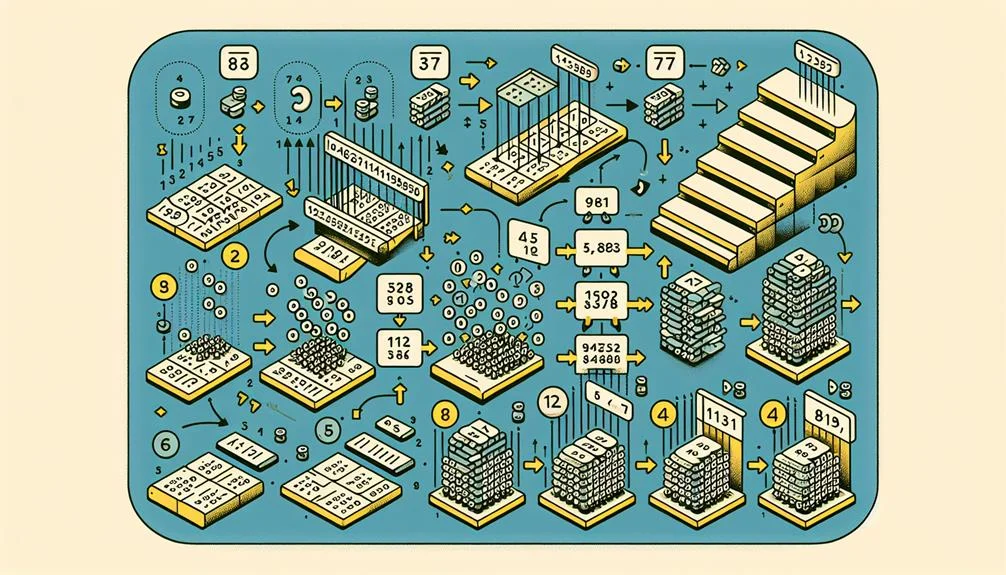
When implementing the Bubble Sort algorithm, the sequence in which elements are sorted plays a critical role in its efficiency and performance.
In Bubble Sort, the algorithm compares adjacent elements in the data and swaps them if they are in the wrong order. The correct position for larger elements is achieved as they gradually ‘bubble up‘ through the array.
The inner loop of the Bubble Sort algorithm iterates through the list multiple times, ensuring that each pass correctly positions the elements until the entire array is sorted.
This step-by-step process of comparing and swapping elements is fundamental to the working of the Bubble Sort algorithm. Understanding the sorting sequence is essential for grasping how Bubble Sort operates and the intricacies of its time and space complexity.
Efficiency of Bubble Sort Algorithm
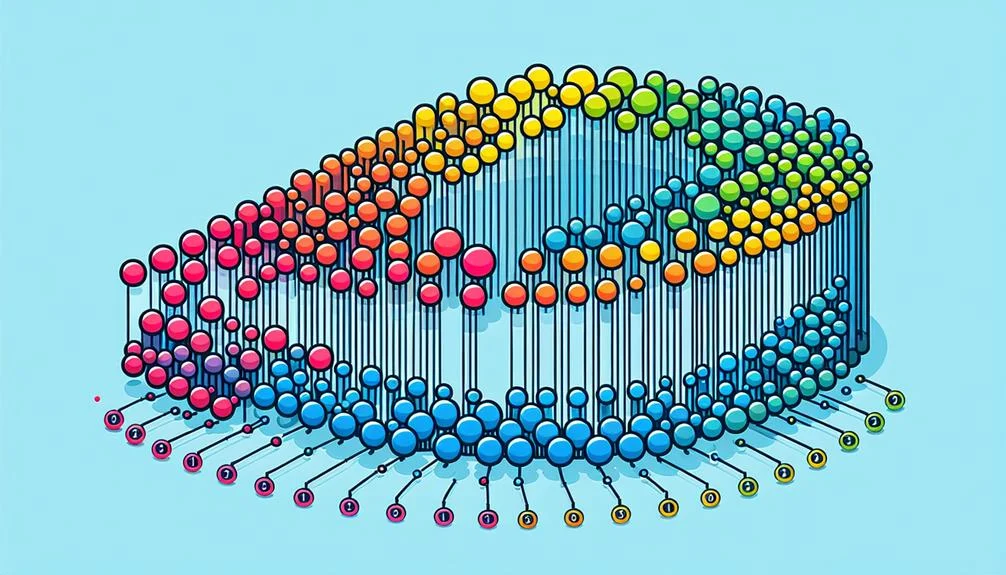
The efficiency of the Bubble Sort algorithm can be analyzed through its time complexity, which is O(n^2) in the worst-case scenario.
Moreover, evaluating the space complexity of Bubble Sort provides insight into its memory usage during sorting operations.
These points are important in understanding the trade-offs involved in using Bubble Sort compared to more efficient sorting algorithms.
Time Complexity Analysis
An analysis of the time complexity of the Bubble Sort algorithm sheds light on its efficiency when sorting data sets.
When considering the time complexity of Bubble Sort, especially when sorting large data sets, several key points come to the forefront:
- Bubble sort exhibits a worst-case time complexity of O(n^2), which can be inefficient when sorting large datasets due to its quadratic time complexity.
- In scenarios where the input is already sorted, Bubble Sort showcases its best-case time complexity of O(n), providing a more efficient sorting process.
- Understanding Bubble Sort’s time complexity as a comparison-based sorting algorithm helps grasp its efficiency in terms of time complexity analysis.
Space Complexity Evaluation
Considering the space complexity of the Bubble Sort algorithm reveals its efficiency in memory utilization. With a space complexity of O(1), bubble sort is designed to operate in-place, sorting elements within the existing array without requiring extra memory proportional to the input size.
This in-place characteristic distinguishes bubble sort from other sorting algorithms that may need supplementary space for temporary storage or auxiliary data structures. The minimal space requirements of bubble sort contribute to its space efficiency, making it a suitable choice in scenarios where optimizing memory usage is vital.
The algorithm’s ability to sort elements within the same array without utilizing extra memory resources enhances its practicality in environments with limited memory availability. Therefore, the space complexity of bubble sort underscores its effectiveness in conserving memory and its applicability in situations where minimizing memory usage is a priority.
Conclusion
To sum up, the Bubble Sort algorithm efficiently sorts elements by systematically comparing and swapping adjacent elements. Through iterative passes, elements gradually move into their correct positions, resulting in a sorted sequence.
However, considering its simplicity and time complexity, one may wonder: Is Bubble Sort the most efficient sorting algorithm for large datasets?