The distinction between Bubble Sort and Quick Sort is pivotal when considering sorting algorithm performance characteristics. While Bubble Sort adopts a simplistic approach of comparing adjacent elements, Quick Sort’s utilization of a strategic pivot selection and divide-and-conquer methodology sets it apart markedly. The efficiency disparity between the two algorithms, especially concerning speed and scalability, reveals a compelling narrative that highlights the intricate nuances of sorting methodologies.
Key Takeaways
- Quick Sort has O(n log n) complexity, outperforming Bubble Sort’s O(n^2) complexity.
- Quick Sort strategically selects pivots, enhancing efficiency compared to Bubble Sort.
- Quick Sort’s divide-and-conquer approach improves sorting speed for large datasets.
- Bubble Sort’s quadratic complexity leads to inefficiencies with large datasets.
- Quick Sort’s partitioning and pivot strategy result in faster sorting times and better performance.
Bubble Sort and Quick Sort Efficiency Comparison
In comparing the efficiency of Bubble Sort and Quick Sort algorithms, it is undeniably evident that Quick Sort demonstrates a superior performance. Quick Sort boasts an average time complexity of O(n log n), a significant improvement over Bubble Sort’s O(n^2) complexity.
This efficiency difference becomes particularly pronounced when dealing with large datasets. Bubble Sort’s quadratic time complexity can lead to significant delays in sorting large amounts of data, whereas Quick Sort’s divide-and-conquer approach allows for faster sorting times even with substantial dataset sizes.
Moreover, while Quick Sort may have a worst-case time complexity of O(n^2), this scenario is less likely to be encountered in practical applications, making Quick Sort a more reliable choice for efficiently sorting large datasets.
Understanding these efficiency disparities is essential when selecting the appropriate sorting algorithm based on the dataset size and performance requirements. Quick Sort’s ability to handle large datasets efficiently positions it as a preferred choice over Bubble Sort in scenarios where time complexity and performance are critical factors to take into account.
Bubble Sort and Quick Sort Approach Differences
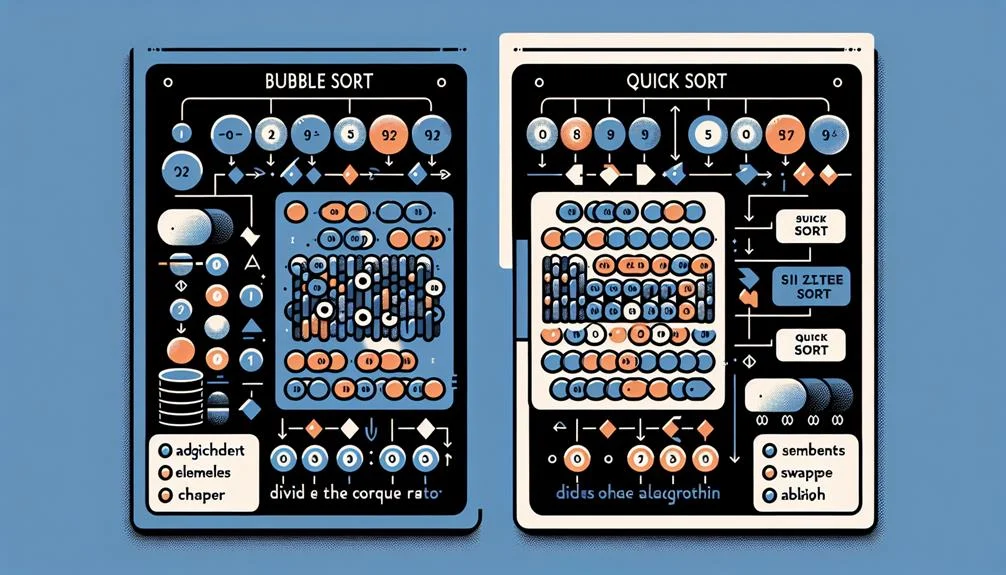
When comparing the approach differences between Bubble Sort and Quick Sort algorithms, two key points come to light: the method of pivot selection and the number of comparisons made during the sorting process.
Quick Sort strategically selects a pivot element for partitioning, optimizing the division of the array. In contrast, Bubble Sort simply compares and swaps adjacent elements in each pass, leading to a higher number of comparisons overall.
Pivot Selection Methods
When it comes to pivot selection in sorting algorithms like Quick Sort and Bubble Sort, the approach differences have a significant impact on determining the efficiency and performance of the sorting process.
Quick Sort’s efficiency heavily relies on the method chosen for pivot selection. The selection of the pivot element, whether it be the first element, a random element, or the median of the array, directly affects the time complexity and sorting speed of the algorithm. By strategically choosing the pivot, Quick Sort can achieve peak performance by efficiently dividing the array into sub-arrays for sorting.
On the other hand, Bubble Sort lacks a pivot selection method, leading to a less efficient sorting process compared to Quick Sort. The absence of a pivot strategy in Bubble Sort contributes to its lower efficiency and performance when sorting large datasets, highlighting the importance of pivot selection in enhancing sorting algorithms’ effectiveness.
Number of Comparisons
Statistically, the number of comparisons required in Bubble Sort surpasses that of Quick Sort, primarily due to their contrasting approaches in sorting elements.
Bubble Sort’s iterative pairwise comparison method leads to a higher comparison count, especially as the input size increases, resulting in a quadratic growth in the number of comparisons needed.
In contrast, Quick Sort’s divide-and-conquer strategy and efficient partitioning allow for a lower comparison count compared to Bubble Sort. By recursively dividing the dataset based on a chosen pivot element, Quick Sort minimizes the number of comparisons required to sort the elements effectively.
Understanding this distinction in comparison counts emphasizes the importance of selecting the most suitable sorting algorithm based on the dataset’s characteristics to optimize efficiency and performance in sorting operations.
Sorting Speed Variation
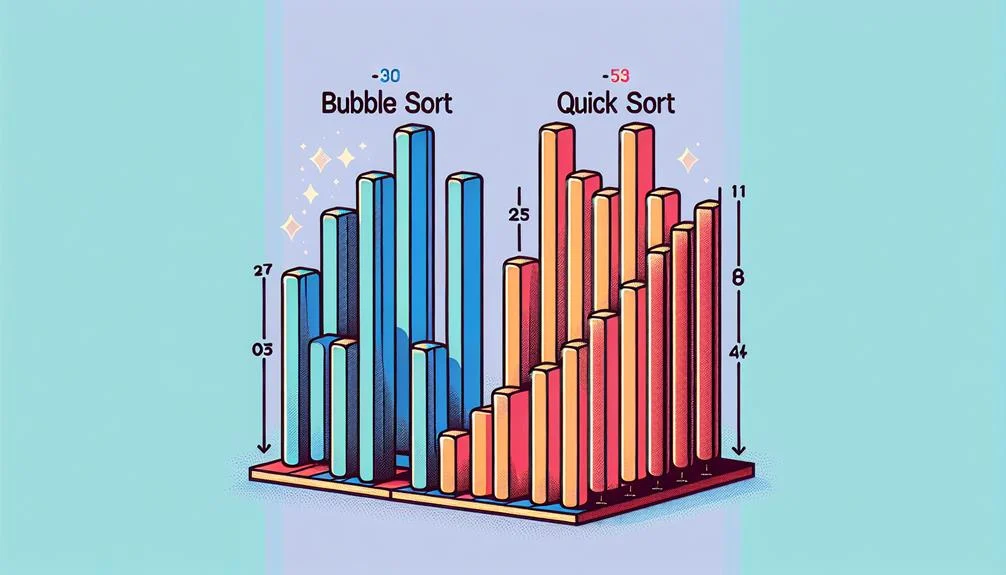
The sorting speed variation between bubble sort and quick sort lies in their efficiency and performance.
Bubble sort exhibits decreasing efficiency as dataset size increases due to its quadratic time complexity, resulting in slower sorting speeds, especially with extensive or unsorted data.
In contrast, quick sort’s divide-and-conquer approach offers faster performance with an average time complexity of O(n log n), making it the preferred choice for larger datasets.
Bubble Sort Efficiency
Bubble Sort’s efficiency with regards to sorting speed variation is greatly impacted by its quadratic time complexity in the worst and average cases. With a time complexity of O(n^2), Bubble Sort becomes increasingly inefficient as the dataset size grows.
This quadratic relationship means that the sorting speed of Bubble Sort decreases notably as the number of elements to be sorted increases. In contrast, Quick Sort‘s time complexity of O(n log n) enables it to efficiently handle large datasets with improved sorting performance.
Quick Sort’s divide-and-conquer strategy and smart pivot selection allow it to outperform Bubble Sort in terms of sorting speed and efficiency. Hence, when dealing with substantial amounts of data, opting for Quick Sort over Bubble Sort can lead to substantial enhancements in sorting performance, reducing processing time and improving overall efficiency in sorting operations.
Quick Sort Performance
When considering sorting speed variation, Quick Sort‘s average time complexity of O(n log n) greatly outperforms Bubble Sort‘s quadratic time complexity, especially for larger datasets.
Quick Sort’s divide-and-conquer approach allows it to consistently perform efficiently with an average time complexity of O(n log n), making it faster and more reliable than Bubble Sort, which has a time complexity of O(n^2) in all cases.
While Quick Sort may have a worst-case scenario of O(n^2), it is less common and generally faster than Bubble Sort. Quick Sort’s performance shines particularly on larger datasets, where its efficient algorithmic design enables quicker sorting compared to Bubble Sort.
In contrast, Bubble Sort’s speed variations become more pronounced as dataset sizes increase, highlighting Quick Sort’s ability to maintain a more consistent and efficient performance across different data sizes.
Impact on Large Datasets
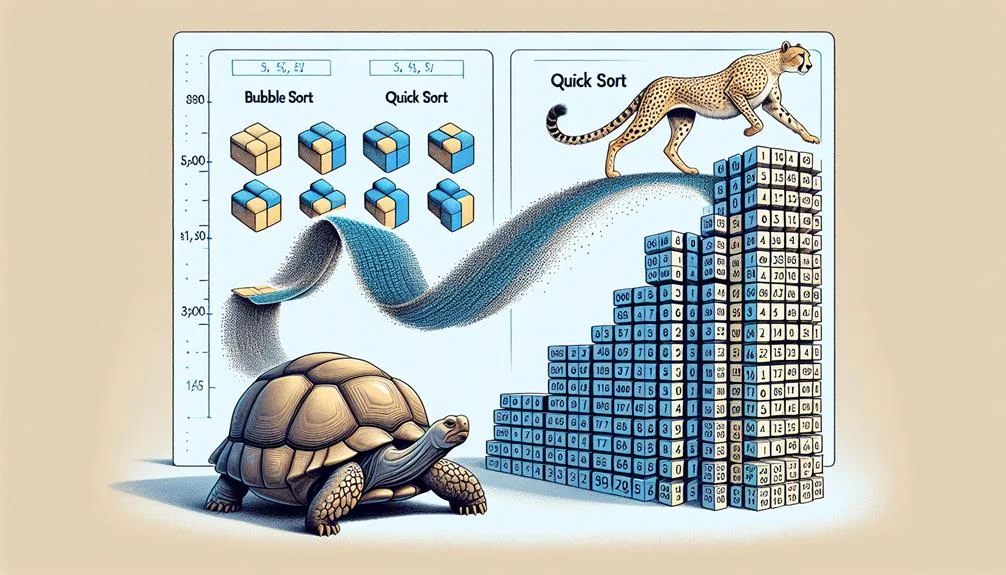
In considerations of sorting algorithms, the efficiency variance between bubble sort and quick sort becomes quite pronounced when handling large datasets.
Quick sort, with its average time complexity of O(n log n), outperforms bubble sort, which has a time complexity of O(n^2), especially as the input size increases.
Quick sort’s divide-and-conquer approach allows it to efficiently sort large datasets by recursively dividing the dataset into smaller sub-arrays and sorting them based on a chosen pivot element.
On the other hand, bubble sort’s performance deteriorates noticeably with larger datasets due to its quadratic time complexity growth.
Opting for quick sort over bubble sort when dealing with large datasets can lead to significantly reduced sorting times and improved overall algorithm efficiency.
Therefore, when faced with sorting tasks involving substantial amounts of data, selecting quick sort proves to be a more effective choice for optimizing sorting time and performance.
Divide and Conquer Concept
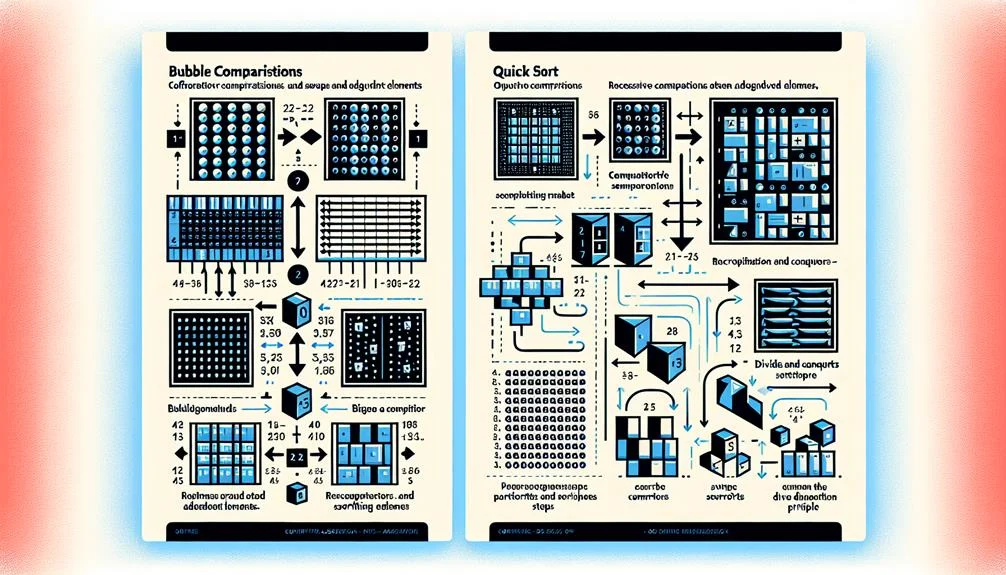
Utilizing the divide and conquer strategy, quick sort efficiently sorts arrays by recursively partitioning them into smaller sub-arrays based on a chosen pivot element. This recursive division and partitioning allow quick sort to achieve higher efficiency in sorting performance, especially with larger datasets, compared to bubble sort’s pairwise comparison approach. The divide and conquer concept in quick sort enables faster sorting by strategically selecting a pivot element, dividing the array into sub-arrays, and then recursively sorting each sub-array. On the other hand, bubble sort sequentially compares adjacent elements and swaps them if they are out of order, lacking the efficient partitioning mechanism of quick sort. The table below illustrates the key differences between the divide and conquer strategy of quick sort and the pairwise comparison approach of bubble sort:
Quick Sort | Bubble Sort |
---|---|
Utilizes divide and conquer strategy | Compares adjacent elements sequentially |
Efficiently sorts through recursive division | Lacks efficient partitioning mechanism |
Fast sorting performance on larger datasets | Slower performance, particularly with larger datasets |
Pivot Selection Importance
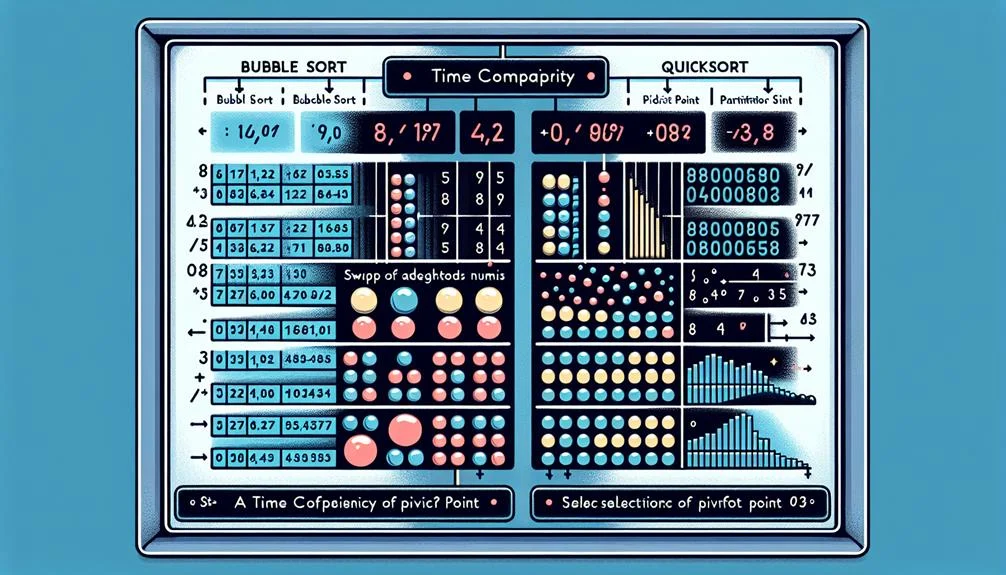
The important role of selecting an ideal pivot element in the Quick Sort algorithm greatly influences its sorting efficiency and performance. The pivot selection directly impacts the division of elements into smaller and larger sublists, affecting the number of comparisons and swaps required for sorting.
Efficient pivot selection leads to a balanced division of elements, enhancing the algorithm’s speed and overall performance. A critical selection of the pivot ensures fast sorting by enabling Quick Sort to efficiently partition the input array. The pivot’s position plays a crucial role in determining the algorithm’s effectiveness in achieving a sorted sequence.
As such, the process of selecting the pivot element is a fundamental aspect of Quick Sort, as it significantly influences the division process, comparisons made, and the overall efficiency of the sorting algorithm. A well-chosen pivot contributes to a more balanced division of elements, ultimately enhancing the speed and performance of the Quick Sort algorithm.
Algorithm Complexity Analysis
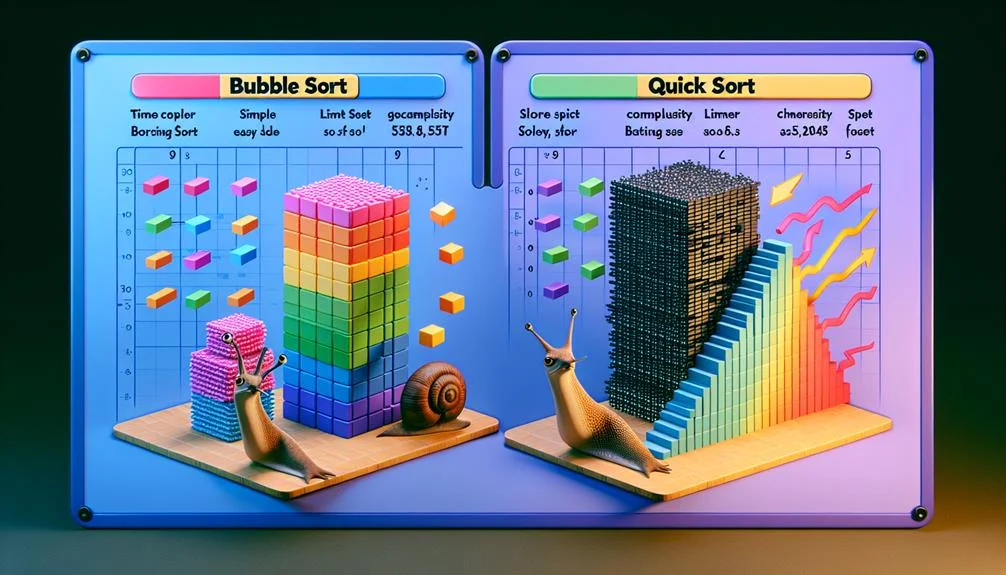
Analyzing the algorithmic complexity of Bubble Sort and Quick Sort reveals significant differences in their efficiency and performance characteristics. Bubble Sort, with its worst-case time complexity of O(n^2), becomes inefficient as the number of elements increases, making it less suitable for sorting large datasets.
In contrast, Quick Sort boasts an average time complexity of O(n log n), making it faster and more efficient for larger datasets compared to Bubble Sort. Bubble Sort typically requires fewer comparisons and swaps in the best-case scenario when the elements are already sorted or nearly sorted, while Quick Sort’s worst-case time complexity of O(n^2) is less common and usually arises from poor pivot selection strategies.
Understanding these complexity differences is important for selecting the appropriate sorting algorithm based on the dataset size and characteristics, ensuring ideal efficiency and performance in various scenarios.
Conclusion
In summary, the efficiency disparity between Bubble Sort and Quick Sort is striking, with Quick Sort’s strategic approach and pivot selection vastly outperforming Bubble Sort’s simplistic comparison method.
The divide-and-conquer strategy of Quick Sort greatly reduces sorting time, especially with large datasets.
The importance of pivot selection cannot be overstated, as it plays a vital role in enhancing the algorithm’s performance.
Quick Sort’s superior efficiency and complexity make it a standout choice for sorting large datasets.